Senior Block Documentation
version 1.1.27
Flight Commands
takeoff()
Block
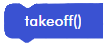
Description
This function makes the CoDrone take off from the ground.
Parameters
None
Returns
None
Example
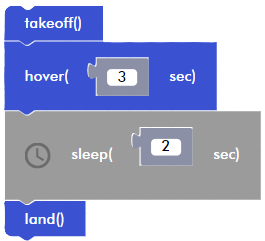
land()
Block

Description
This function lands the CoDrone slowly.
Parameters
None
Returns
None
Example
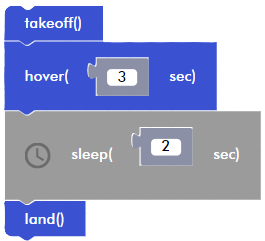
emergency_stop()
Block
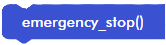
Description
Stops all commands to motors. The CoDrone will stop flying immediately.
Parameters
None
Returns
None
Example
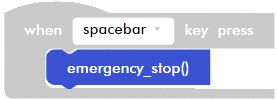
move([duration] sec, [roll]%, [pitch]%, [yaw]%, [throttle]%)
Block

Description
Moves CoDrone based on the given roll, pitch, yaw, and throttle power for a given duration, in seconds.
Parameters
duration: time of movement/flight
roll: the power for the roll movement (-100 - 100)
pitch: the power for the pitch movement (-100 - 100)
yaw: the power for the yaw movement (-100 - 100)
throttle: the power for the throttle (-100 - 100)
Returns
None
Example
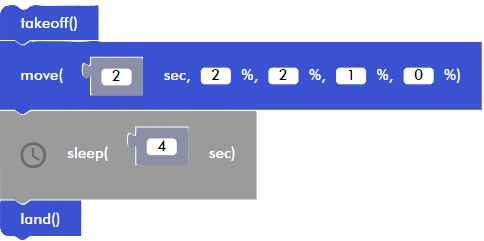
turn([direction], [duration] seconds, [power]%)
Block

Description
Turns the CoDrone to a given direction for a given duration, in seconds, at a given power/speed.
Parameters
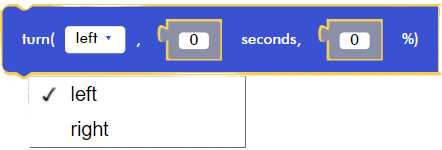
direction: the direction of the turn
duration: the duration of the turn
power: the power/speed of the turn
Returns
None
Example
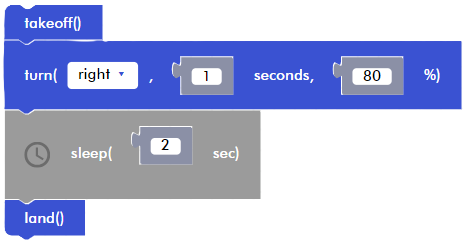
hover([duration] sec)
Block
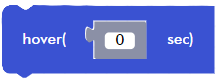
Description
Makes the CoDrone hover for a given duration, in seconds.
Parameters
duration: duration of the hover
Returns
None
Example
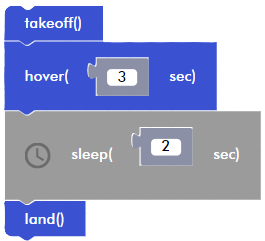
Flight Variables
set_roll([power]%)
Block
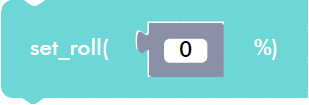
Description
This function sets the roll direction variable but will not move the CoDrone. Negative values will move the drone to the left and positive values will move the drone to the right.
Parameters
power: integer between -100 and 100
Returns
None
Example
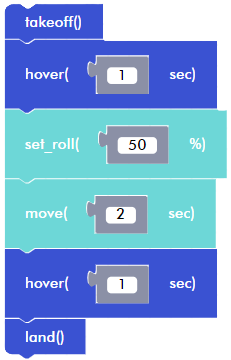
set_pitch([power]%)
Block
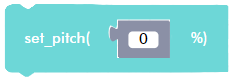
Description
This function sets the pitch direction variable but will not move the CoDrone. Negative values will move the drone to the backward and positive values will move the drone to the forward.
Parameters
power: integer between -100 and 100
Returns
None
Example
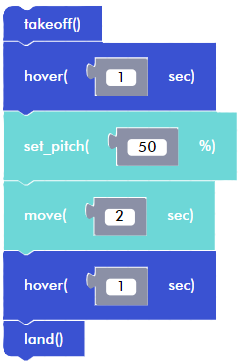
set_yaw([power]%)
Block
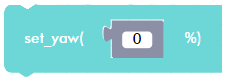
Description
This function sets the yaw direction variable but will not rotate the CoDrone. Negative values will rotate the drone to the left and positive values will rotate the drone to the right.
Parameters
power: integer between -100 and 100
Returns
None
Example
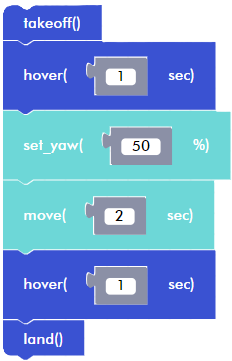
set_throttle([power]%)
Block
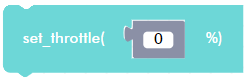
Description
This function sets the throttle direction variable but will not move the CoDrone. Negative values will move the drone to the down and positive values will move the drone up.
Parameters
power: integer between -100 and 100
Returns
None
Example
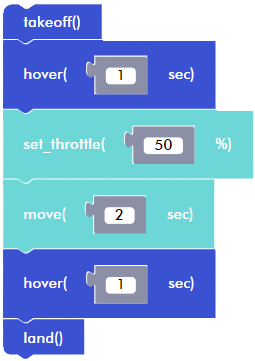
move([duration] sec)
Block
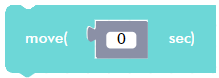
Description
Moves the CoDrone for a given duration based on the flight variable blocks preceding this block.
Parameters
duration: the time of the movement
Returns
None
Example
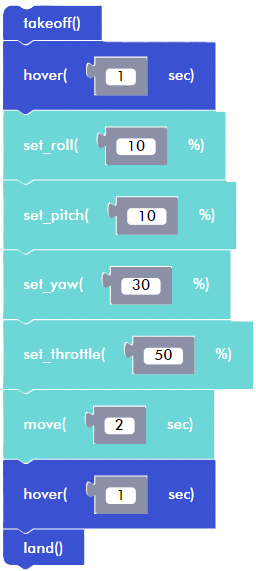
move()
Block
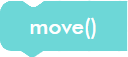
Description
Moves the CoDrone indefinitely based on the flight variable blocks preceding this block.
Parameters
None
Returns
None
Example
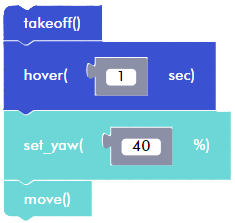
get_roll()
Block
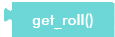
Description
Returns the current value for the roll flight variable.
Parameters
None
Returns
roll: the power of the roll (-100 - 100)
Example
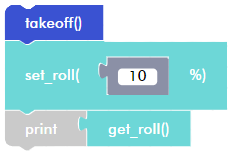
get_pitch()
Block
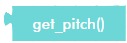
Description
Returns the current value for the pitch flight variable.
Parameters
None
Returns
pitch: the power of the pitch (-100 - 100)
Example
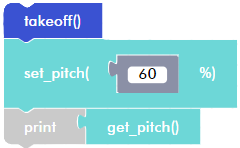
get_yaw()
Block
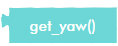
Description
Returns the current value for the yaw flight variable.
Parameters
None
Returns
yaw: the power of the yaw (-100 - 100)
Example
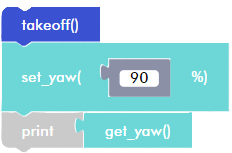
get_throttle()
Block
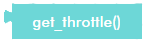
Description
Returns the current value for the throttle flight variable.
Parameters
None
Returns
throttle: the power of the throttle (-100 - 100)
Example
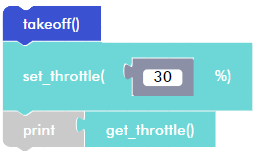
Status Checkers
is_code_running()
Block
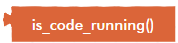
Description
Returns a True value while your code is running. Use this block instead of "while True" when you want to run a "forever" loop. Use the "Stop" button in Blockly to stop the program.
Parameters
None
Returns
None
Example
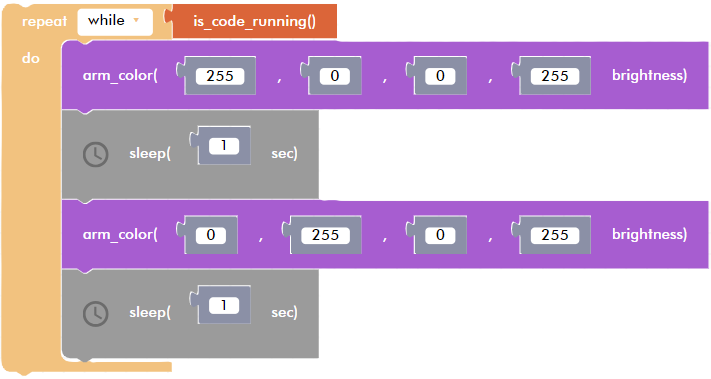
Lights
arm_color([red], [green], [blue], [brightness] brightness)
Block

Description
Sets the LED color of CoDrone's arms using RGB values and their brightness with value from 1 - 100.
Parameters
red: Pixel value for the color red
green: Pixel value for the color green
blue: Pixel value for the color blue
brightness: brightness of the LED color
Returns
None
Example
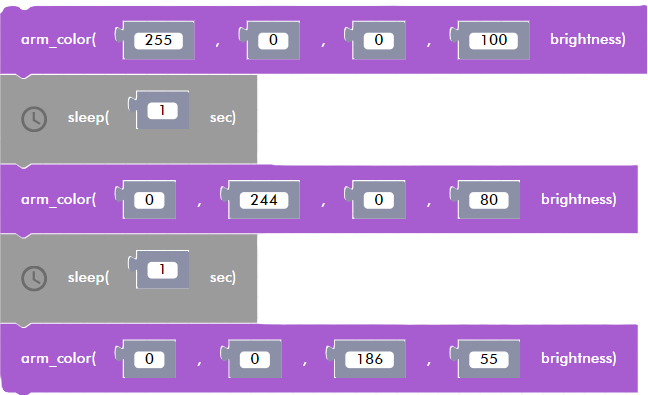
eye_color([red], [green], [blue], [brightness] brightness)
Block

Description
Sets the LED color of CoDrone's eyes using RGB values and their brightness with value from 1 - 100.
Parameters
red: Pixel value for the color red
green: Pixel value for the color green
blue: Pixel value for the color blue
brightness: brightness of the LED color
Returns
None
Example
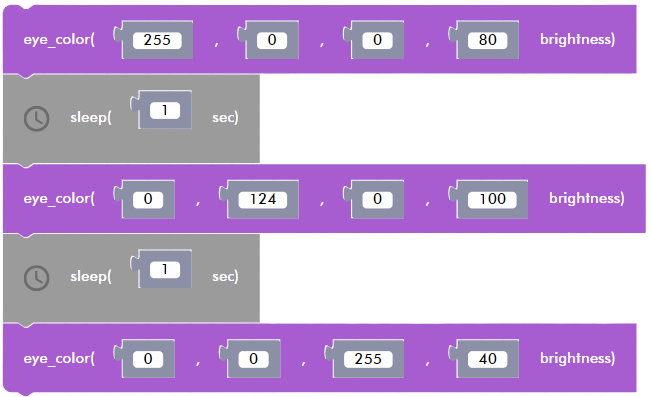
arm_default_color([red], [green], [blue], [brightness] brightness)
Block

Description
Sets the default LED color of CoDrone's arms using RGB values and their brightness with value from 1 - 100, so it will remain that color even after powering off and back on.
Parameters
red: Pixel value for the color red
green: Pixel value for the color green
blue: Pixel value for the color blue
brightness: brightness of the LED color
Returns
None
Example

eye_default_color([red], [green], [blue], [brightness] brightness)
Block

Description
Sets the default LED color of CoDrone's eyes using RGB values and their brightness with value from 1 - 100, so it will remain that color even after powering off and back on.
Parameters
red: Pixel value for the color red
green: Pixel value for the color green
blue: Pixel value for the color blue
brightness: brightness of the LED color
Returns
None
Example

arm_pattern([red], [green], [blue], [pattern], [speed] speed)
Block

Description
Sets the LED color of CoDrone's arms using RGB values with a given pattern at a given speed
Parameters
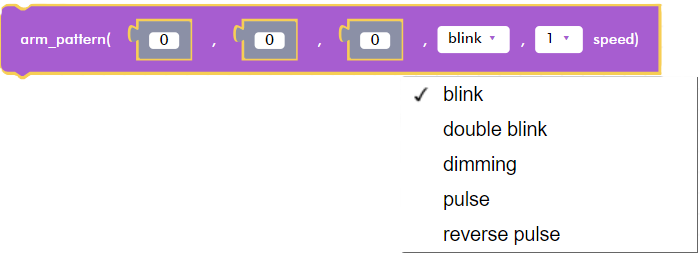
red: pixel value for the color red
green: pixel value for the color green
blue: pixel value for the color blue
pattern: lighting pattern of the LEDs
speed: speed of the lighting pattern
Returns
None
Example
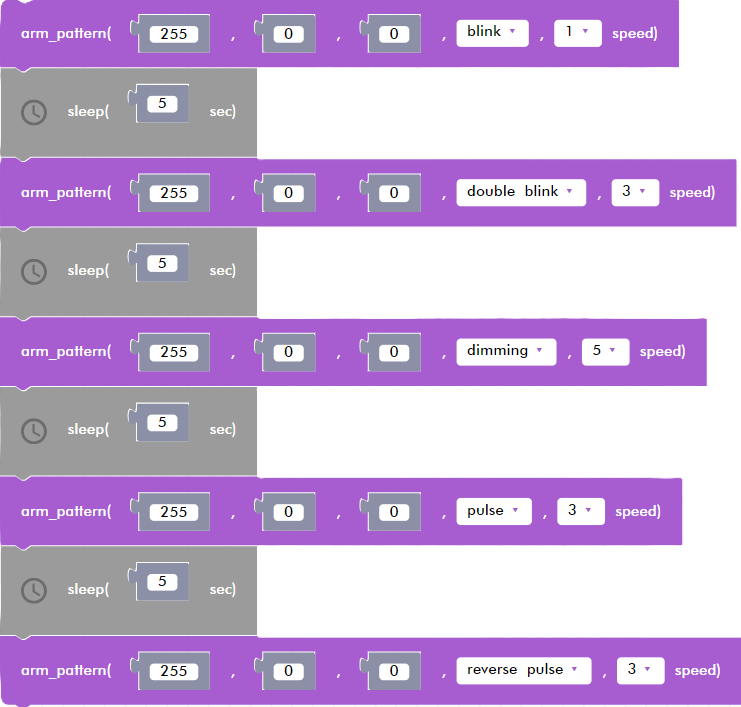
eye_pattern([red], [green], [blue], [pattern], [speed] speed)
Block

Description
Sets the LED color of CoDrone's eyes using RGB values with a given pattern at a given speed
Parameters
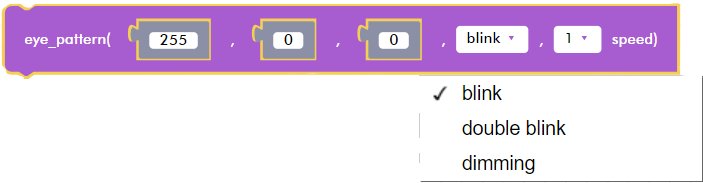
red: pixel value for the color red
green: pixel value for the color green
blue: pixel value for the color blue
pattern: lighting pattern of the LEDs
speed: speed of the lighting pattern
Returns
None
Example
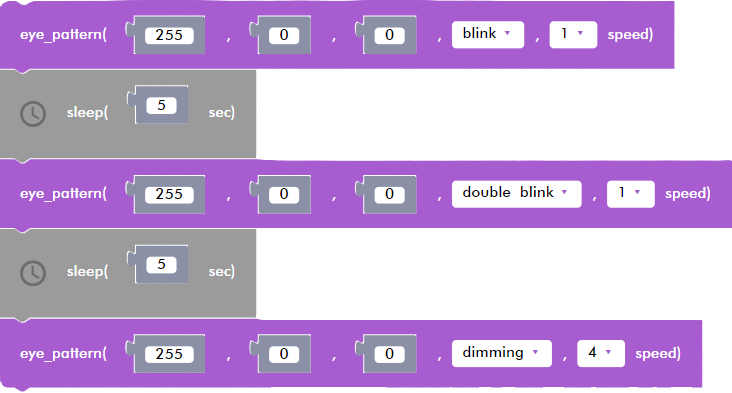
default_eye_pattern([red], [green], [blue], [pattern], [speed] speed)
Block

Description
Sets default LED color of CoDrone's eyes using RGB values with a given pattern at a given speed, which means the lights will remain in that color and pattern when powered off and back on.
Parameters
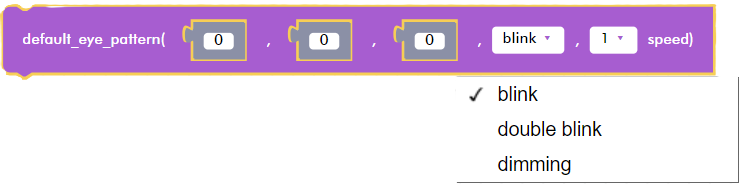
red: pixel value for the color red
green: pixel value for the color green
blue: pixel value for the color blue
pattern: lighting pattern of the LEDs
speed: speed of the lighting pattern
Returns
None
Example

default_arm_pattern([red], [green], [blue], [pattern], [speed] speed)
Block

Description
Sets default LED color of CoDrone's arms using RGB values with a given pattern at a given speed, which means the lights will remain in that color and pattern when powered off and back on.
Parameters
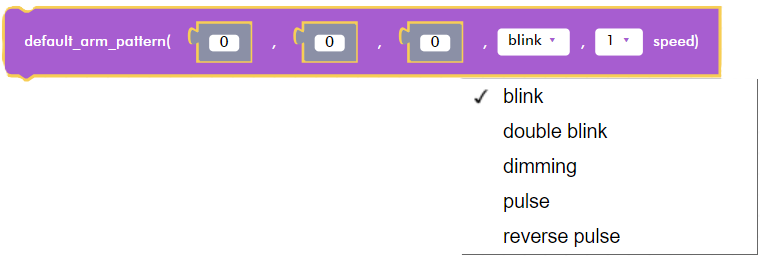
red: pixel value for the color red
green: pixel value for the color green
blue: pixel value for the color blue
pattern: lighting pattern of the LEDs
speed: speed of the lighting pattern
Returns
None
Example

arm_strobe()
Block
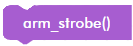
Description
CoDrone turns on strobing effect for arm lights.
Parameters
None
Returns
None
Example
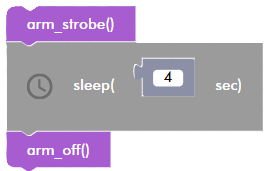
eye_strobe()
Block
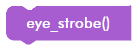
Description
CoDrone turns on strobing effect for eye lights.
Parameters
None
Returns
None
Example
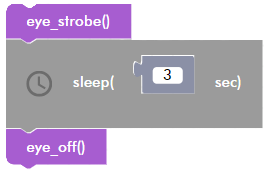
arm_default_strobe()
Block
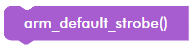
Description
Sets a default strobing effect for arm lights, which will still be in effect after it's turned off and on.
Parameters
None
Returns
None
Example
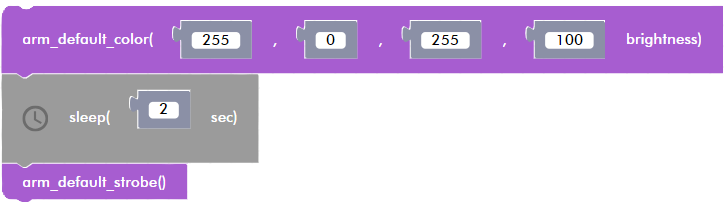
eye_default_strobe()
Block
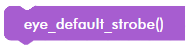
Description
Sets a default strobing effect for eye lights, which will still be in effect after it's turned off and on.
Parameters
None
Returns
None
Example
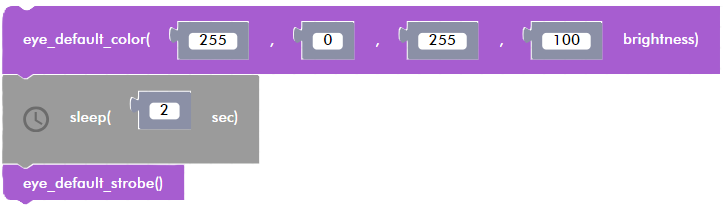
arm_off()
Block
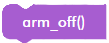
Description
Turns off all arm lights off.
Parameters
None
Returns
None
Example
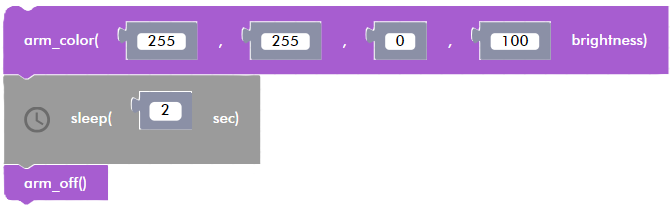
eye_off()
Block
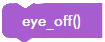
Description
Turns off all eye lights off.
Parameters
None
Returns
None
Example
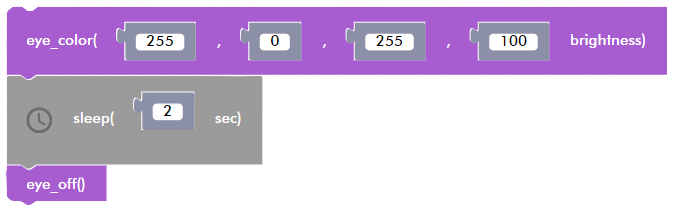