Junior Block Documentation
version 2.92
Driving
forward
Block
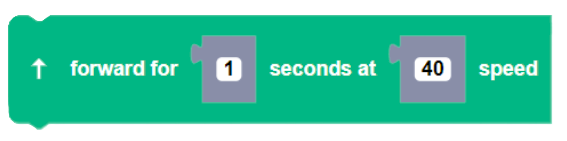
Description
Moves Zumi forward for the given duration and speed
Parameters
float seconds: A value, in seconds, for the duration of the movement.
integer speed: A value (0 - 100) for the speed of the movement.
Returns
None
Example
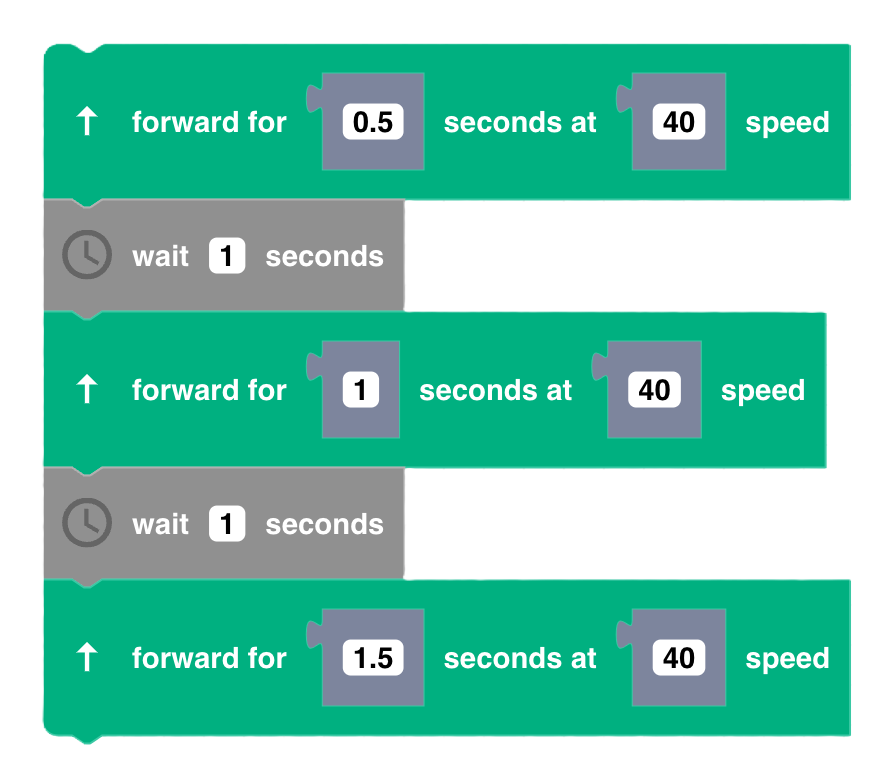
reverse
Block
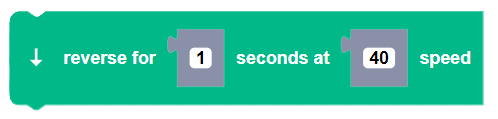
Description
Moves Zumi backwards for the given duration and speed
Parameters
float seconds: A value, in seconds, for the duration of the movement.
integer speed: A value (0 - 100) for the speed of the movement.
Returns
None
Example
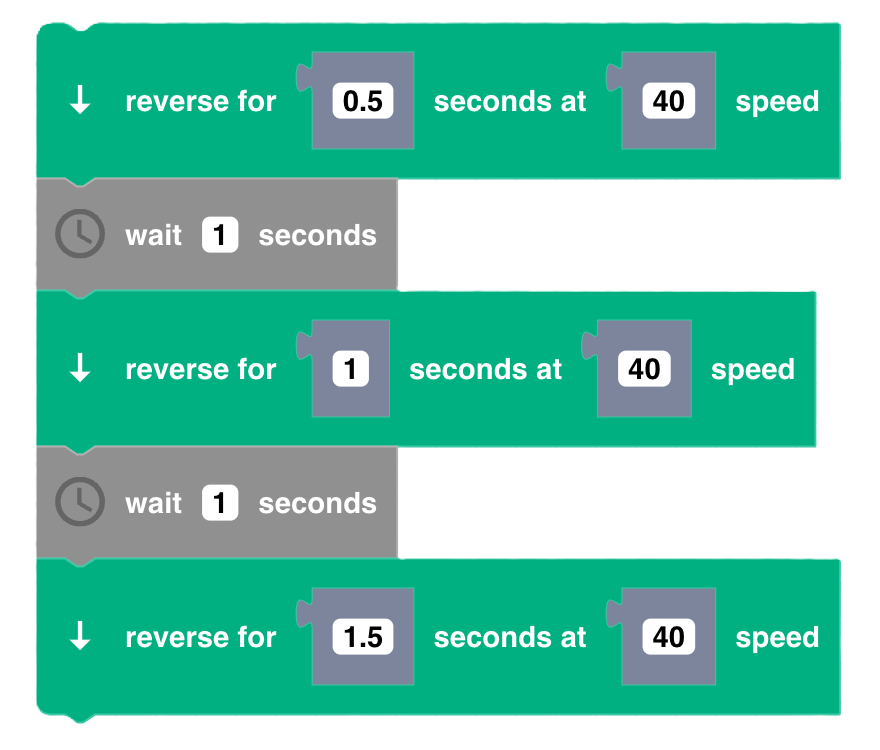
turn left
Block
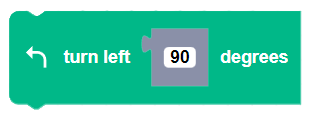
Description
Turns Zumi to the left a number of specified degrees.
Parameters
integer degrees: The angle of the left turn in degrees
Returns
None
Example
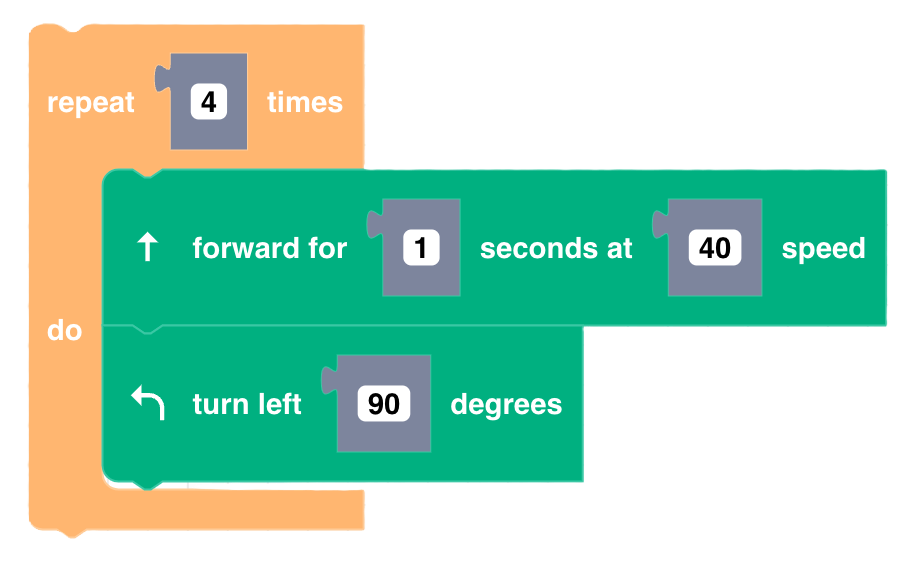
turn right
Block
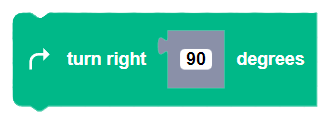
Description
Turns Zumi to the right a number of specified degrees.
Parameters
integer degrees: The angle of the right turn in degrees
Returns
None
Example
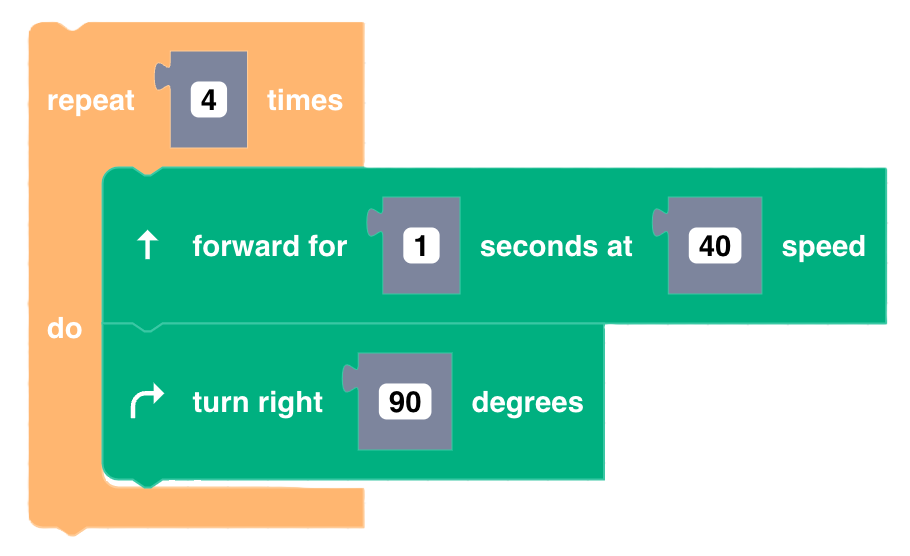
stop
Block
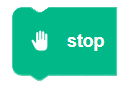
Description
Stops Zumi's motors when running indefinite move commands such as the Senior forward_step() block.
Parameters
None
Returns
None
Example
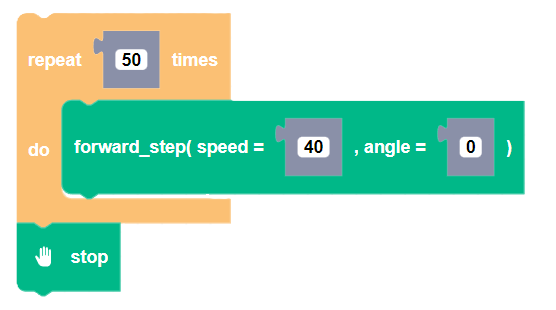
left u turn
Block
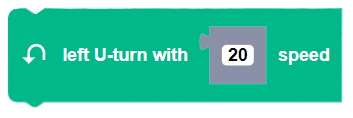
Description
Makes Zumi perform a left "U-turn". As the speed increases, the turn radius gets larger.
Parameters
integer speed: The speed of the U-turn (0 - 100)
Returns
None
Example
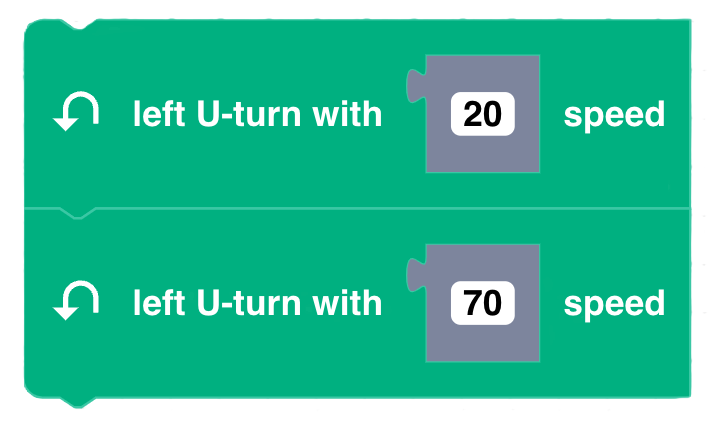
right u turn
Block
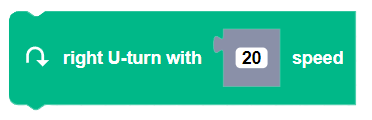
Description
Makes Zumi perform a right "U-turn". As the speed increases, the turn radius gets larger.
Parameters
integer speed: The speed of the U-turn (0 - 100)
Returns
None
Example
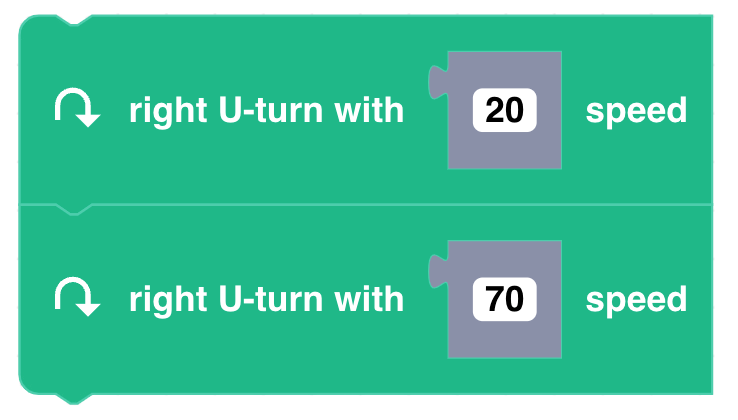
parallel park
Block
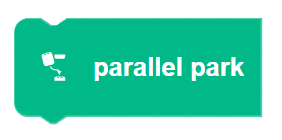
Description
Drives Zumi in reverse into a parallel park.
Parameters
None
Returns
None
Example
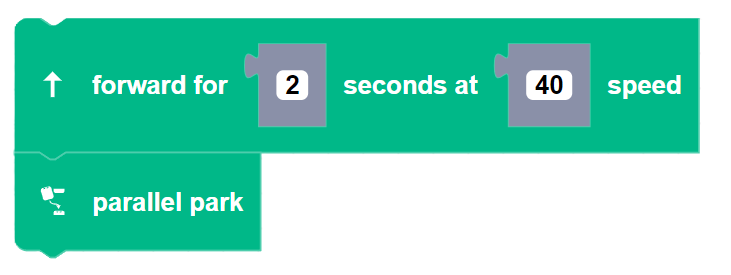
calibrate gyro
Block
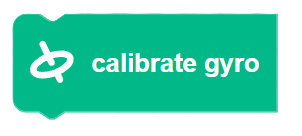
Description
Recalibrates Zumi's gyroscope. Use when the gyroscope returns values with high error due to drift. During recalibration, Zumi should be stationary on a flat surface.
Parameters
None
Returns
None
Example
In this example/scenario, the Zumi wasn't driving straight when the first forward block ran, drifting off to the side. After the calibrate gyro block and the second forward block ran, Zumi was able to drive straight as it should.
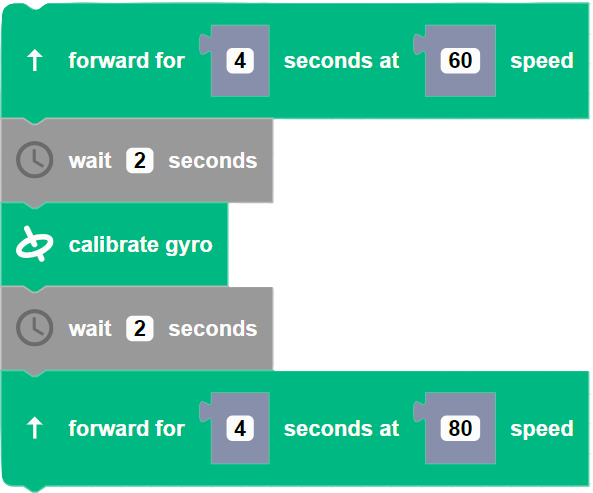
Shapes
triangle
Block
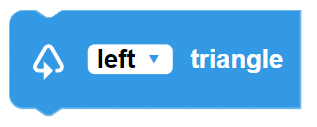
Description
Drives Zumi in the shape of a triangle using either left or right turns.
Parameters
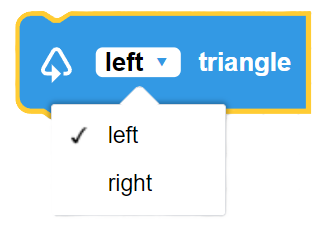
left: Makes a triangle with left turns
right: Makes a triangle with right turns
Returns
None
Example
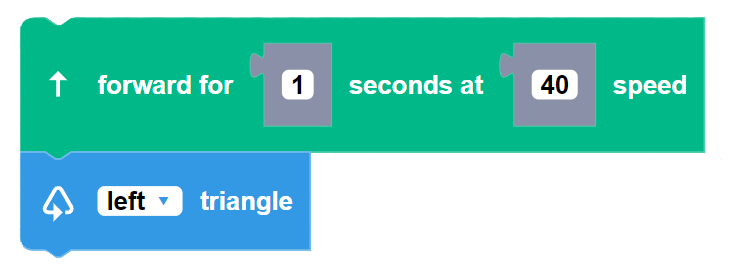
square
Block
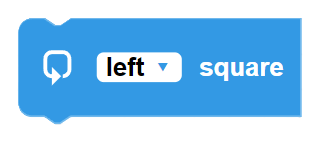
Description
Drives Zumi in the shape of a square using either left or right turns.
Parameters
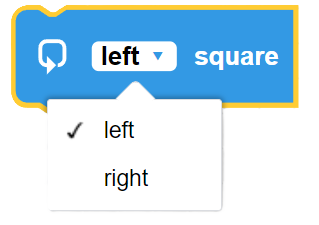
left: Makes a square with left turns.
right: Makes a square with right turns.
Returns
None
Example
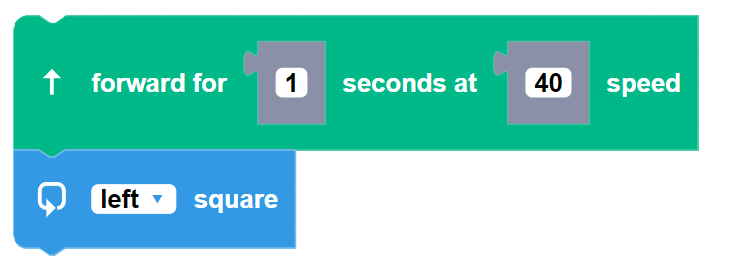
rectangle
Block
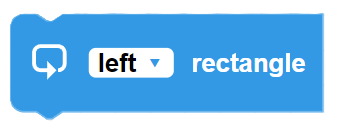
Description
Drives Zumi in the shape of a rectangle using either left or right turns.
Parameters
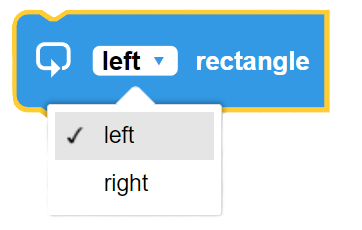
left: Makes a rectangle with left turns.
right: Makes a rectangle with right turns.
Returns
None
Example
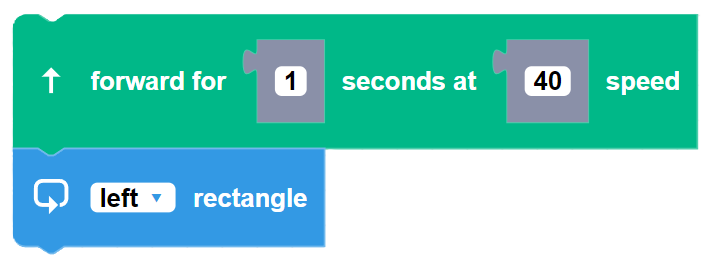
circle
Block
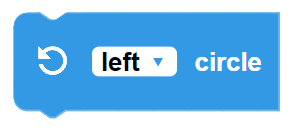
Description
Drives Zumi in the shape of a circle using either left or right turns.
Parameters
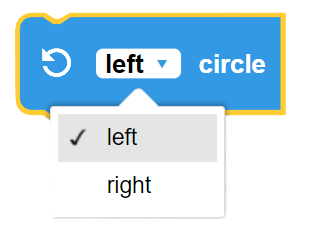
left: Makes a circle turning to the left.
right: Makes a circle turning to the right.
Returns
None
Example
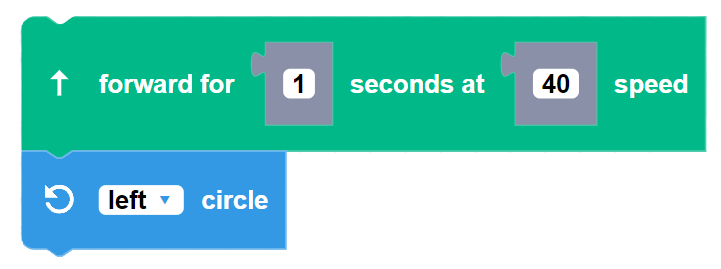
figure 8
Block
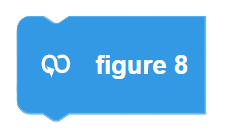
Description
Drives Zumi in the shape of a figure 8. Zumi does a full circle to the right, and then a full circle to the left.
Parameters
None
Returns
None
Example
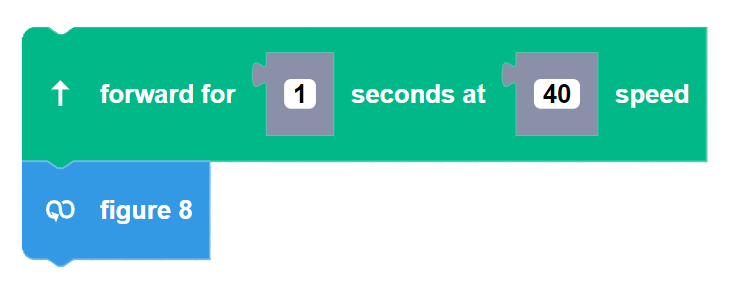
j turn
Block
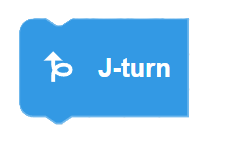
Description
Drives Zumi in the shape of a J turn. Zumi will start by driving forward
Parameters
None
Returns
None
Example
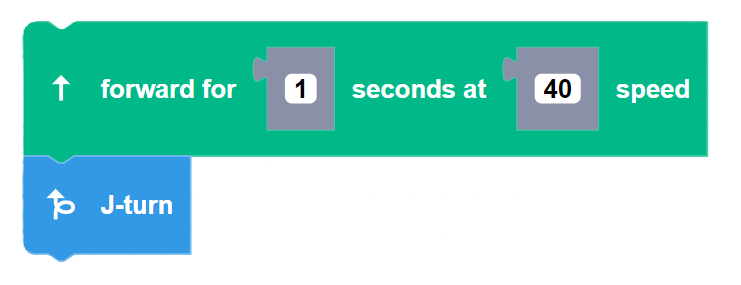
Screen
draw text
Block
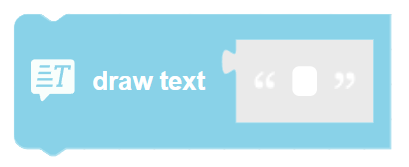
Description
Draws a string of letters and characters and centers them on Zumi's screen. The screen can draw a maximum of three lines of text, each around 14 characters long.
Parameters
string text: Inputted text to display
Returns
None
Example
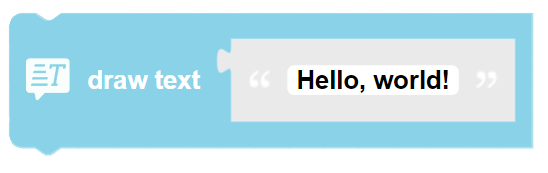
sad
Block
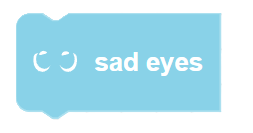
Description
Displays Zumi's sad eyes on the screen.
Parameters
None
Returns
None
Example
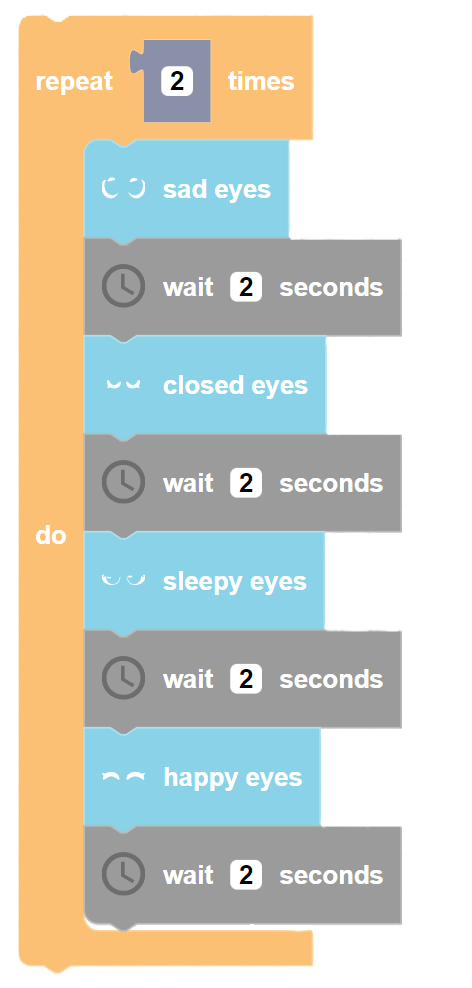
closed
Block
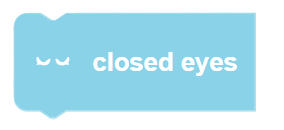
Description
Displays Zumi's closed eyes on the screen.
Parameters
None
Returns
None
Example
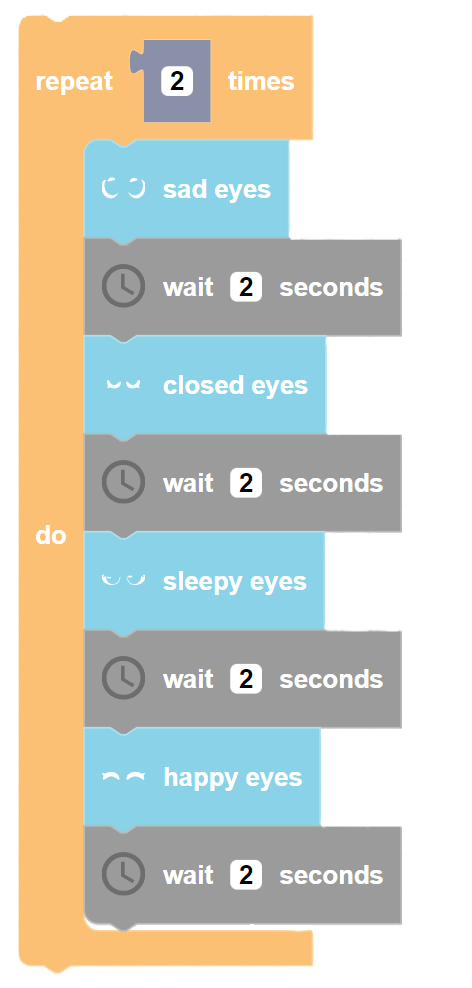
sleepy
Block
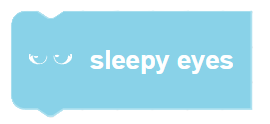
Description
Displays Zumi's sleepy eyes on the screen.
Parameters
None
Returns
None
Example
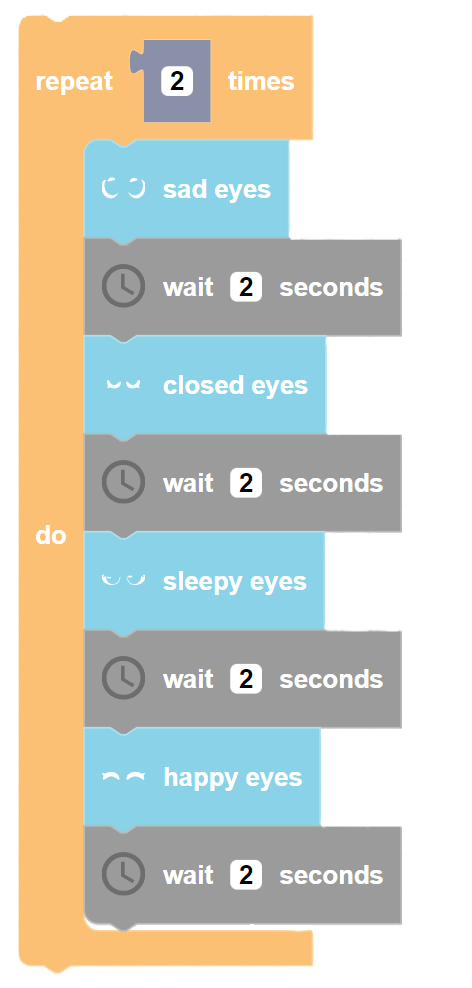
happy
Block
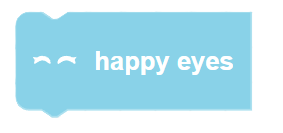
Description
Displays Zumi's happy eye animation on the screen.
Parameters
None
Returns
None
Example
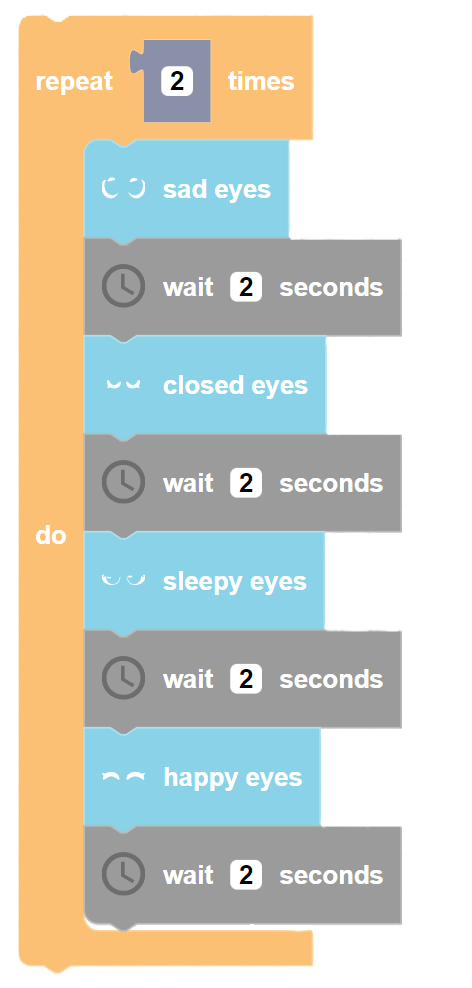
glimmer
Block
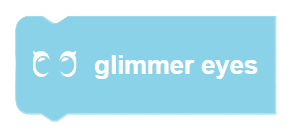
Description
Displays Zumi's glimmer eye animation on the screen.
Parameters
None
Returns
None
Example
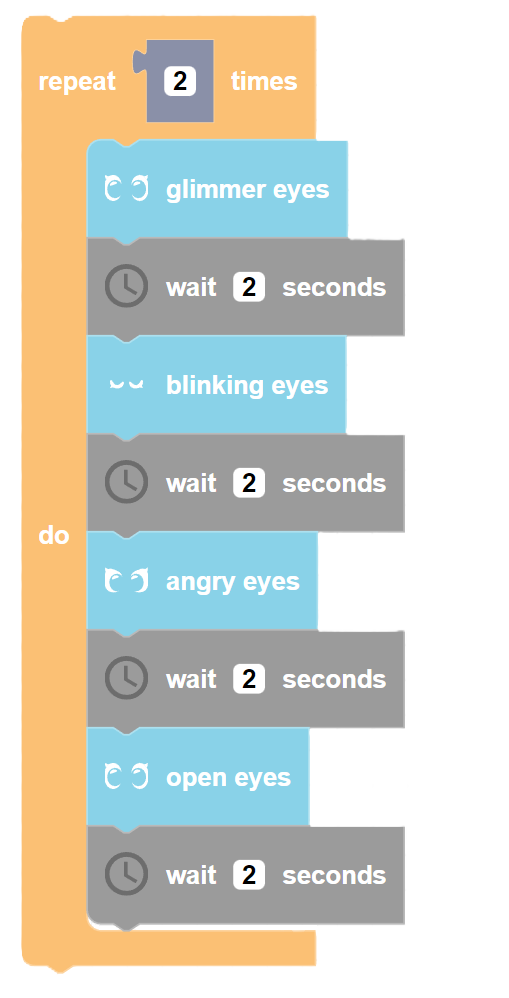
blinking
Block
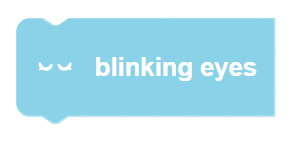
Description
Displays Zumi's blinking eye animation on the screen.
Parameters
None
Returns
None
Example
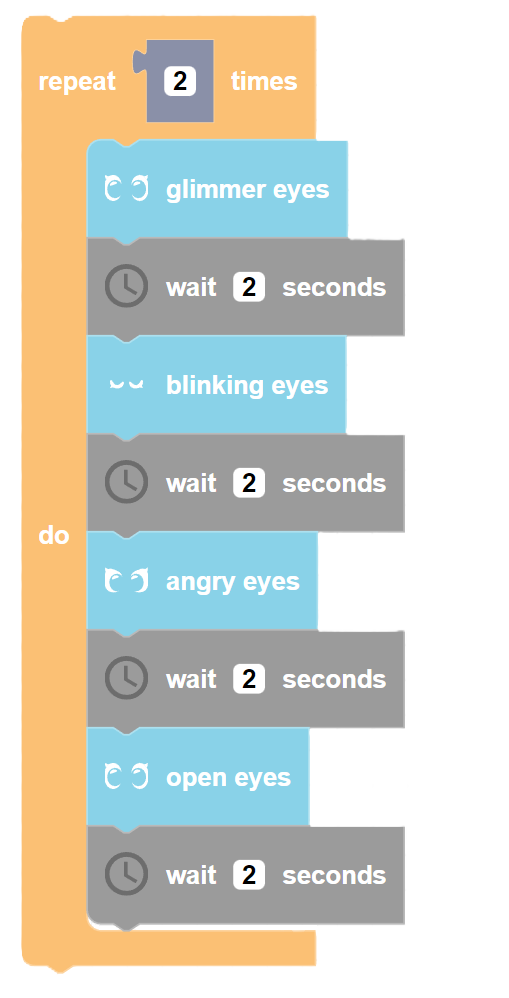
angry
Block
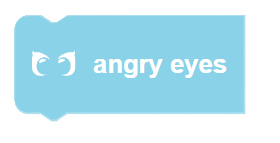
Description
Displays Zumi's angry eyes on the screen.
Parameters
None
Returns
None
Example
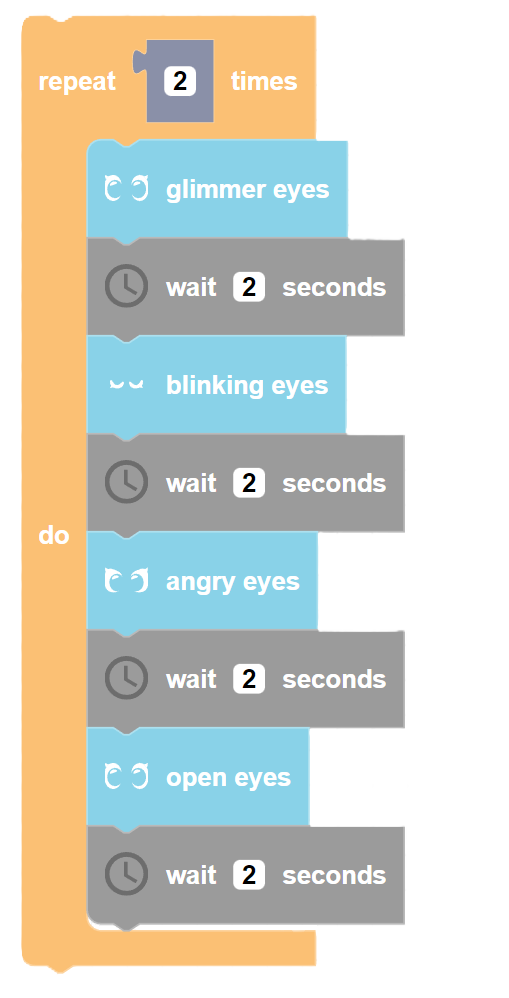
open
Block
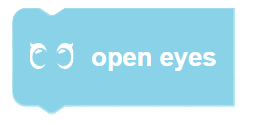
Description
Displays Zumi's open eyes on the screen.
Parameters
None
Returns
None
Example
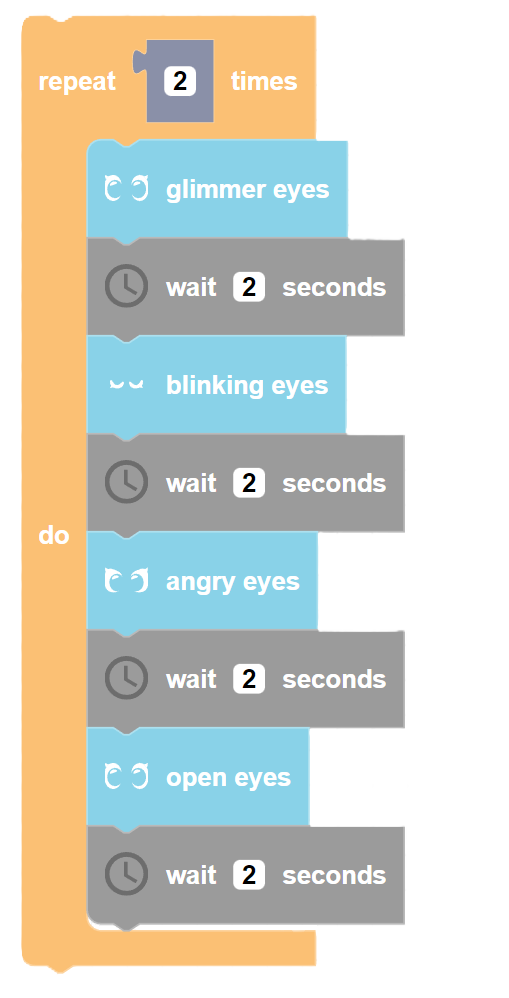
Sounds
play note
Block
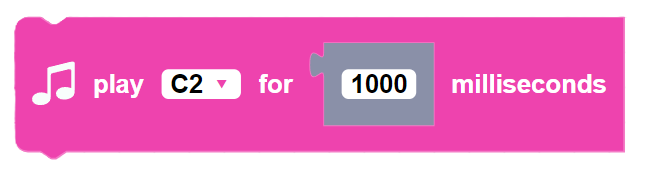
Description
Plays a single note for a specified duration in milliseconds.
Parameters
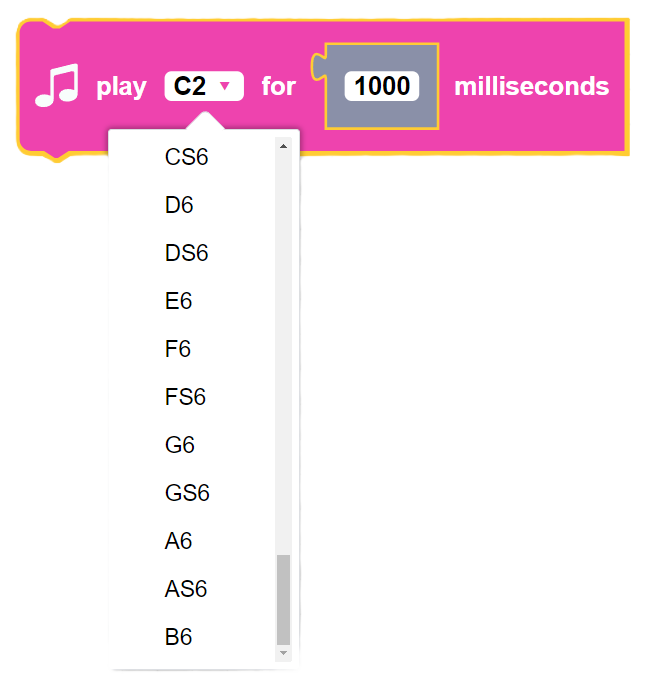
note: The note that is played. Ranging from C2 to B6
integer duration: The duration of note played in milliseconds
Returns
None
Example
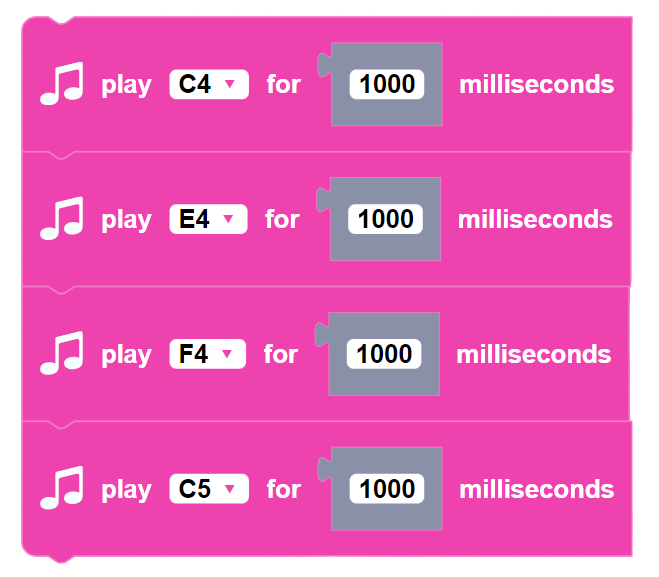
angry
Block
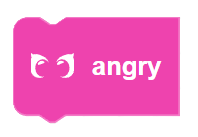
Description
Plays Zumi's angry sound effect.
Parameters
None
Returns
None
Example
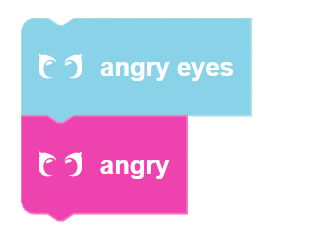
happy
Block
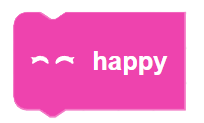
Description
Plays Zumi's happy sound effect.
Parameters
None
Returns
None
Example
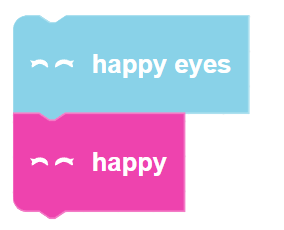
blink
Block
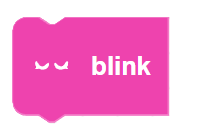
Description
Plays Zumi's blinking sound effect.
Parameters
None
Returns
None
Example
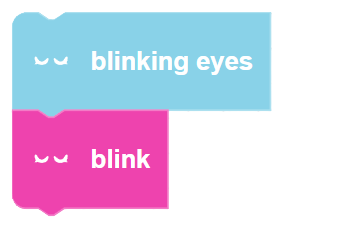
celebrate
Block
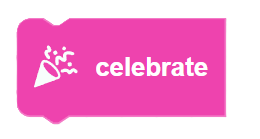
Description
Plays Zumi's celebration sound effect.
Parameters
None
Returns
None
Example
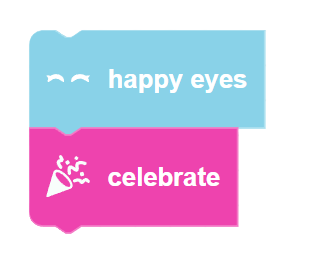
wakeup
Block
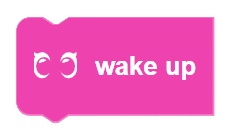
Description
Plays Zumi's wake up sound effect.
Parameters
None
Returns
None
Example
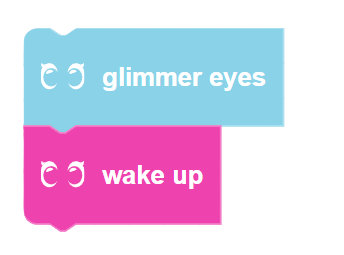
disoriented
Block
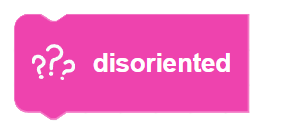
Description
Plays Zumi's disoriented sound effect.
Parameters
None
Returns
None
Example
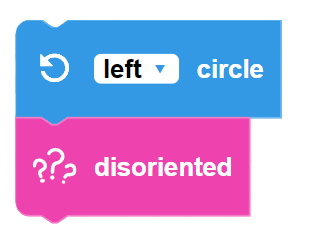
oops front
Block
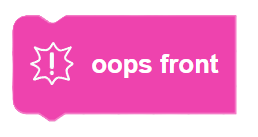
Description
Plays Zumi's front sensor detect sound effect.
Parameters
None
Returns
None
Example
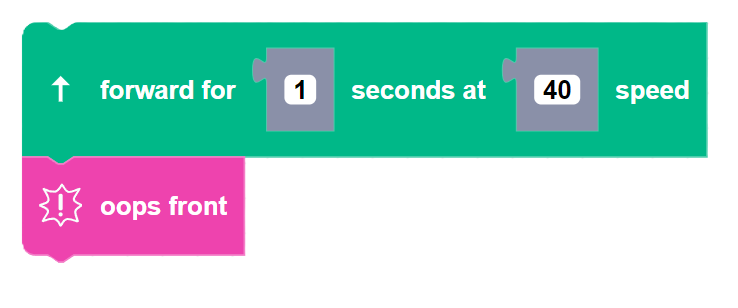
oops back
Block
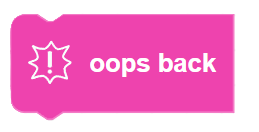
Description
Plays Zumi's back sensor detect sound effect.
Parameters
None
Returns
None
Example
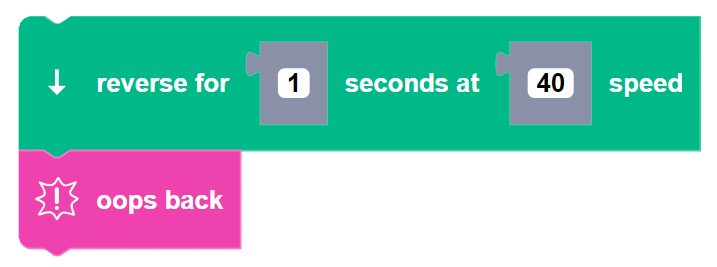
Sensors
get IR reading
Block
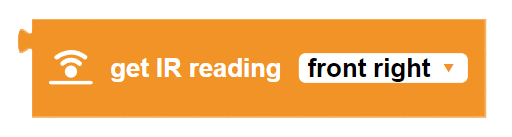
Description
Gets the current IR reading from Zumi's sensors. The closer the value is to 0, the more IR light is being detected. Used commonly when detecting objects since an object or obstacle nearby will reflect IR light back to the receiver.
Parameters
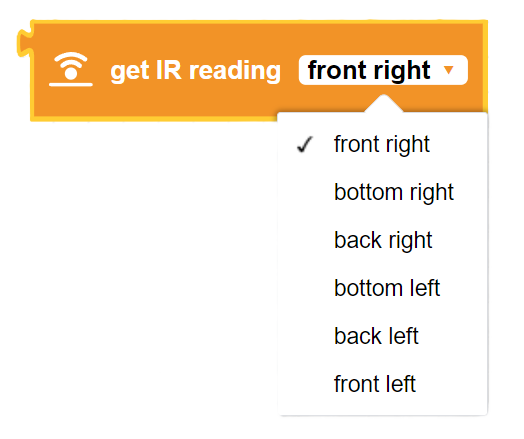
Returns
integer IR sensor value: The amount of IR light being detected by the IR sensor (0 - 255)
Example
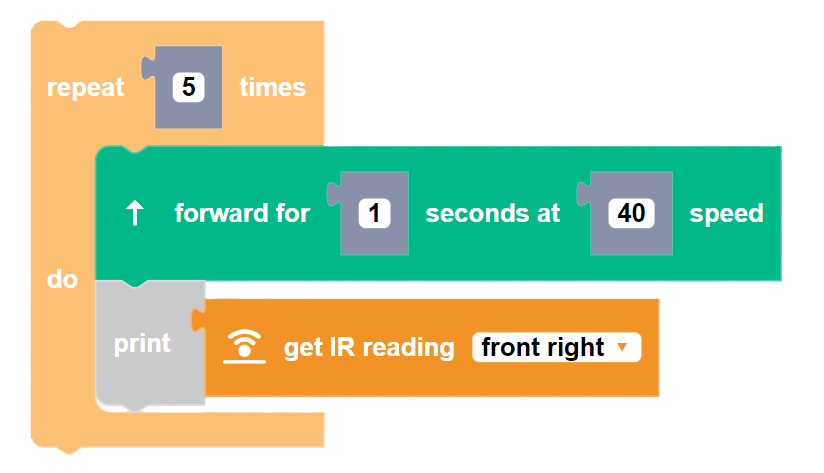
get z angle
Block
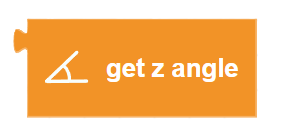
Description
Gets the current Z angle from Zumi's gyroscope.
Parameters
None
Returns
integer angle: The z-angle of the gyroscope reading (0 - 360)
Example
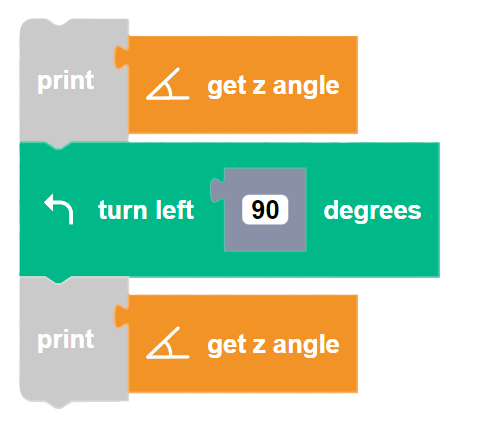
get x angle
Block
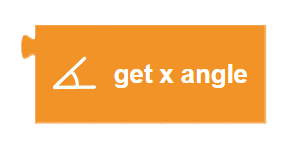
Description
Gets the current X angle from Zumi's gyroscope.
Parameters
None
Returns
integer angle: The x-angle of the gyroscope reading (0 - 360)
Example
With this example, tilt Zumi left and right with your hands to see the X angle change!
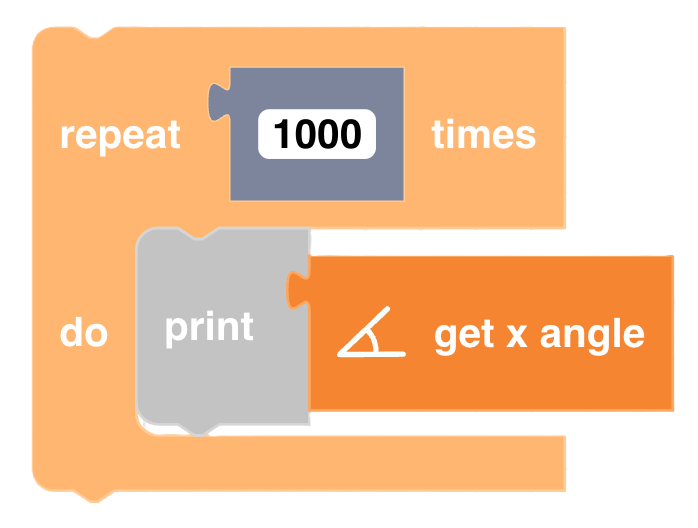
get y angle
Block
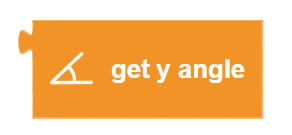
Description
Gets the current Y angle from Zumi's gyroscope.
Parameters
None
Returns
integer angle: The y-angle of the gyroscope reading (0 - 360)
Example
With this example, rock Zumi forward and backward with your hands to see the Y angle change!
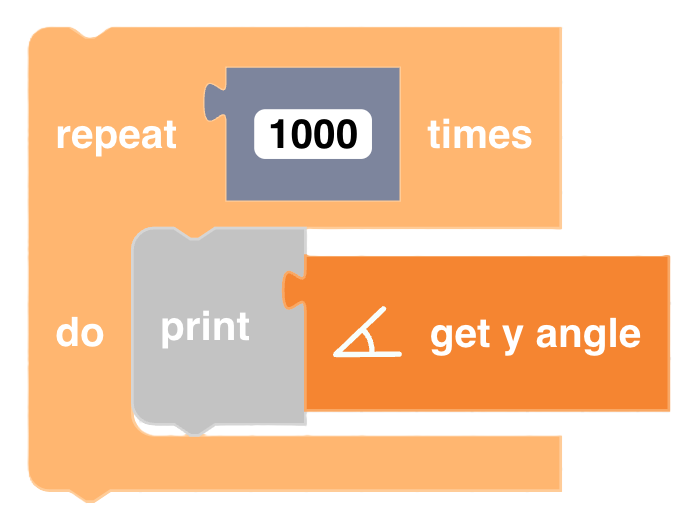
reset gyro
Block
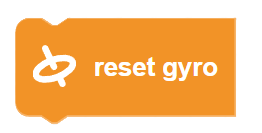
Description
Resets Zumi's gyroscope x, y, and z angles to 0. Use this function to reset Zumi's heading.
Parameters
None
Returns
None
Example
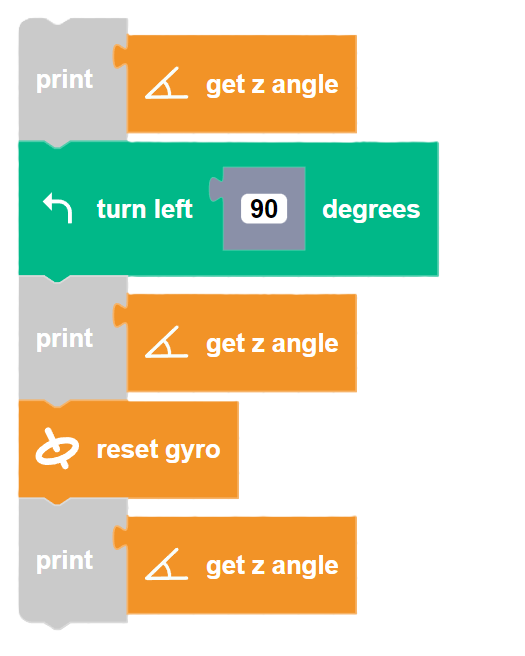
get battery voltage
Block
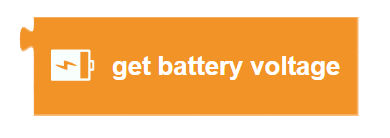
Description
Gets the current voltage from Zumi's battery. It typically varies from 3.45V-4.14V when not connected over USB. If connected over USB, this function may return 1.7V or below.
Parameters
None
Returns
float voltage: The battery's voltage in units of Volts
Example
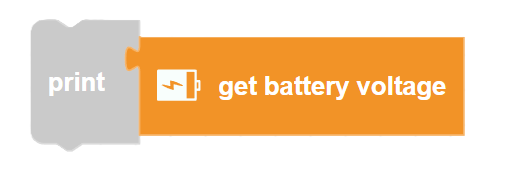
get battery percentage
Block
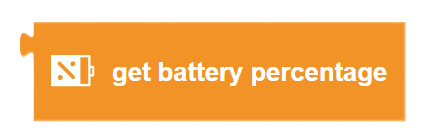
Description
Gets the current battery percentage from Zumi's battery when not connected over USB power. The percentage will vary while driving since it is dependent on battery voltage.
Parameters
None
Returns
integer percent: The battery's percentage (0 - 100)
Example
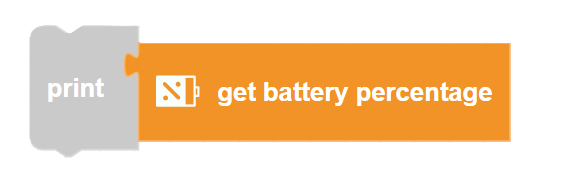
Camera
import camera
Block
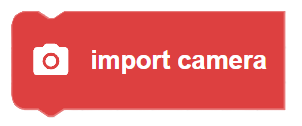
Description
Imports the camera library. This block must be included at the top of any code that uses camera functions.
Parameters
None
Returns
None
Example
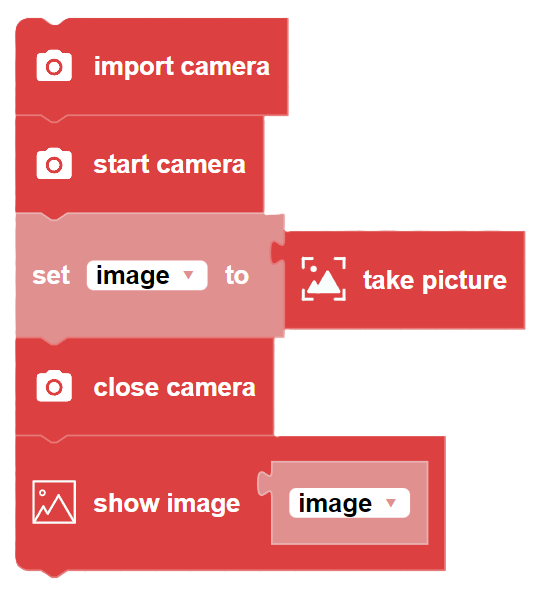
start camera
Block
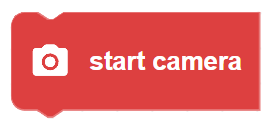
Description
Turns on Zumi's camera. A red LED will turn on next to the camera to indicate the camera is on. The camera needs to turn on before taking any pictures and will stay on until it is manually turned off with the close_camera() block.
Parameters
None
Returns
None
Example
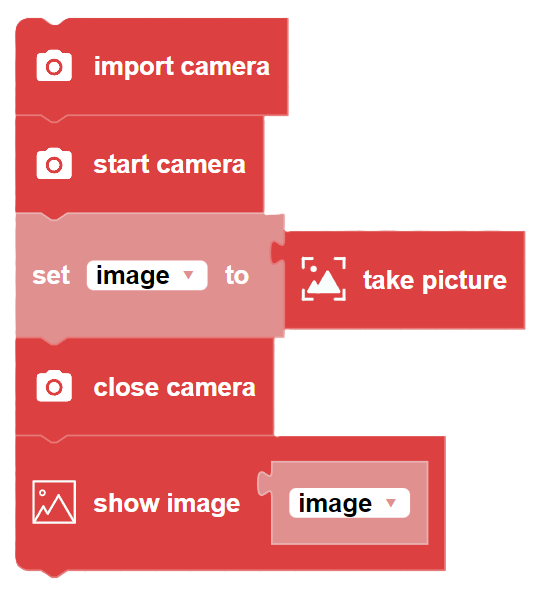
close camera
Block
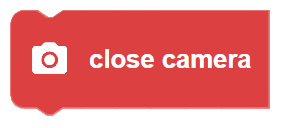
Description
Turns off Zumi's camera. It is recommended to always turn off the camera when not in use to conserve battery. The camera cannot be started again unless the camera is already off.
Parameters
None
Returns
None
Example
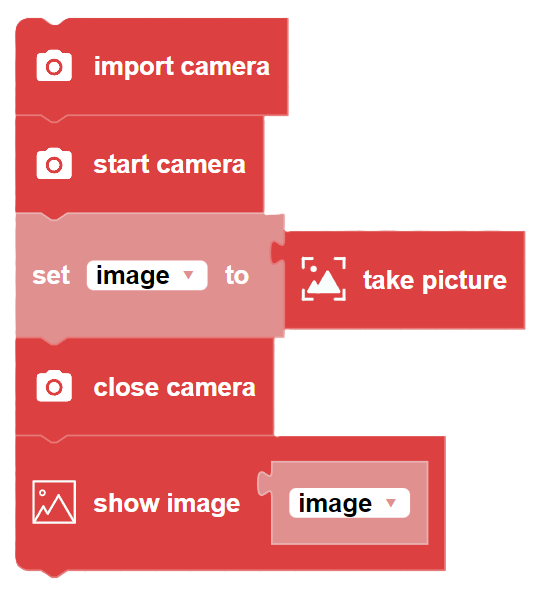
take picture
Block
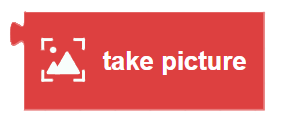
Description
Captures a color image with Zumi's camera and stores the array as a frame object. Use the image object with show_image() to display in Blockly. This block cannot be used without importing and starting the camera.
Parameters
None
Returns
ndarray image: An image composed of a NumPy 160x128 array of pixels.
Example
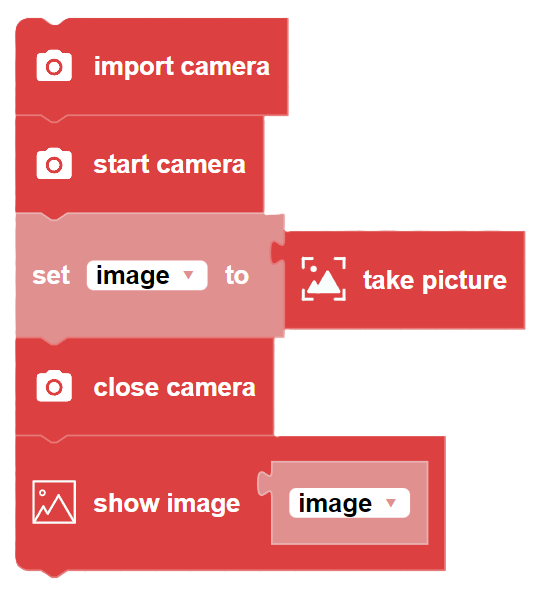
show image
Block
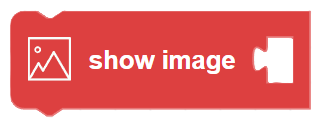
Description
Show an image that was taken with Zumi's camera in Blockly.
Parameters
ndarray image: An image composed of a NumPy array of pixels.
Returns
None
Example
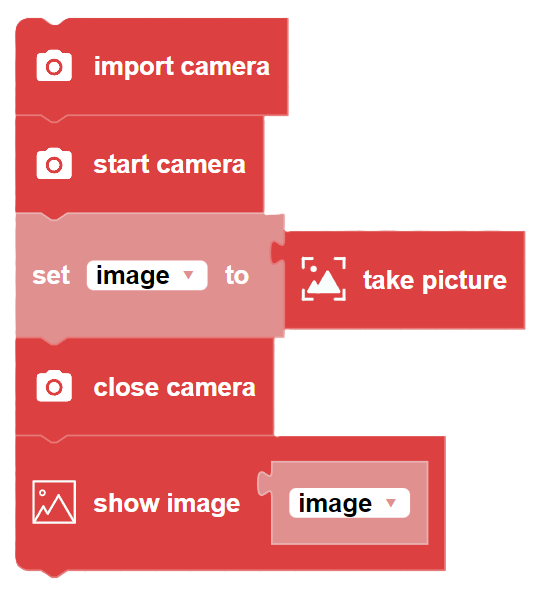
get QR code message
Block
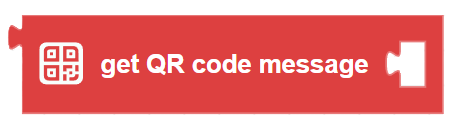
Description
Searches an image for a QR code message. If a QR code was found, the encoded message is saved to a string.
Parameters
ndarray image: An image composed of a NumPy array of pixels.
Returns
string message: The decoded message of the QR code. Returns None
if no QR code was detected.
Example
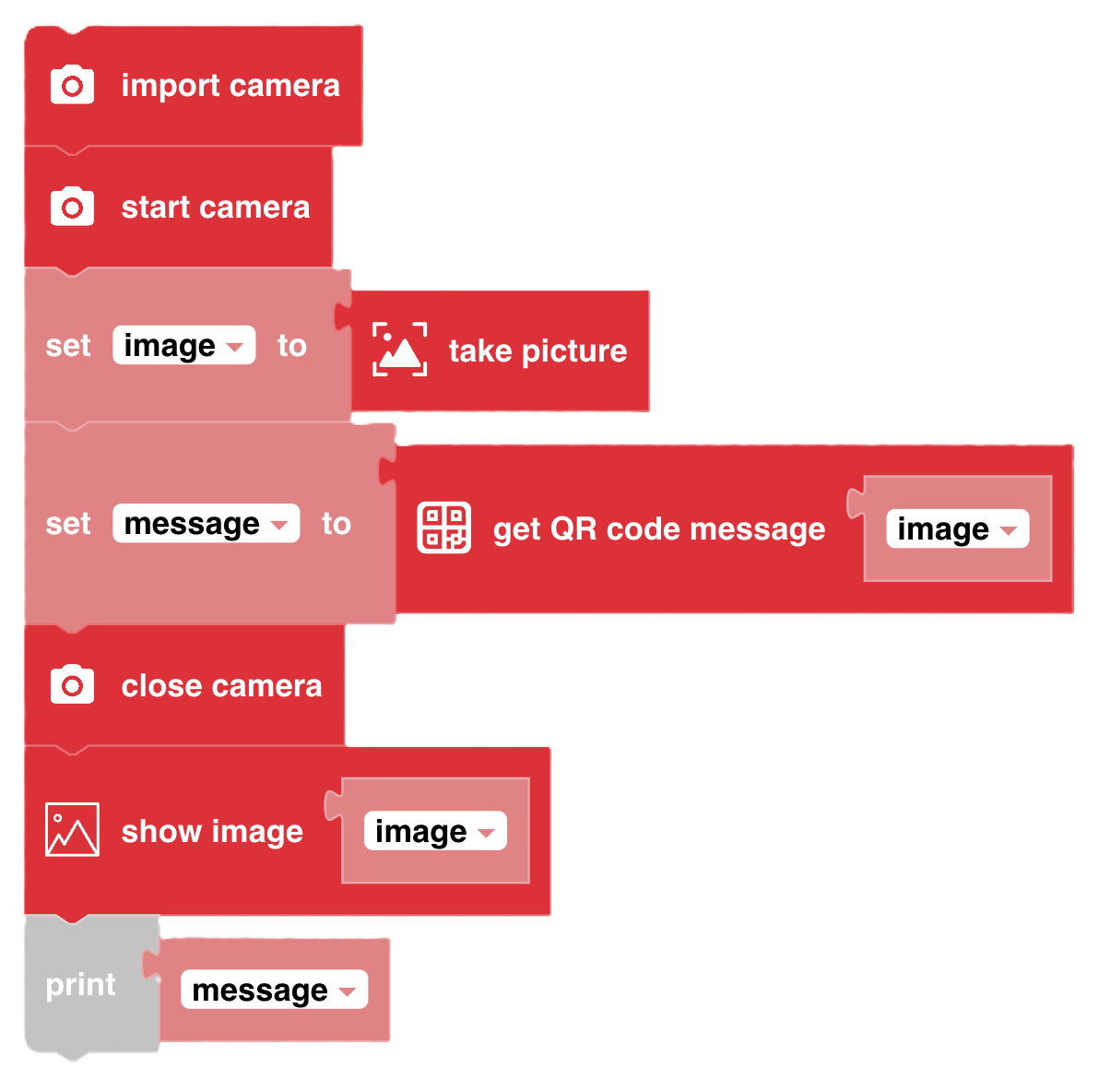
find stop sign
Block
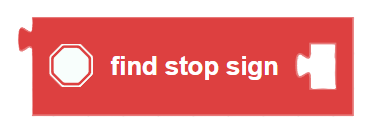
Description
Searches an image for a stop sign. Returns True if a stop sign was found.
Parameters
ndarray image: An image composed of a NumPy array of pixels.
Returns
boolean detection: Returns True if a stop sign was detected. Otherwise, returns False.
Example
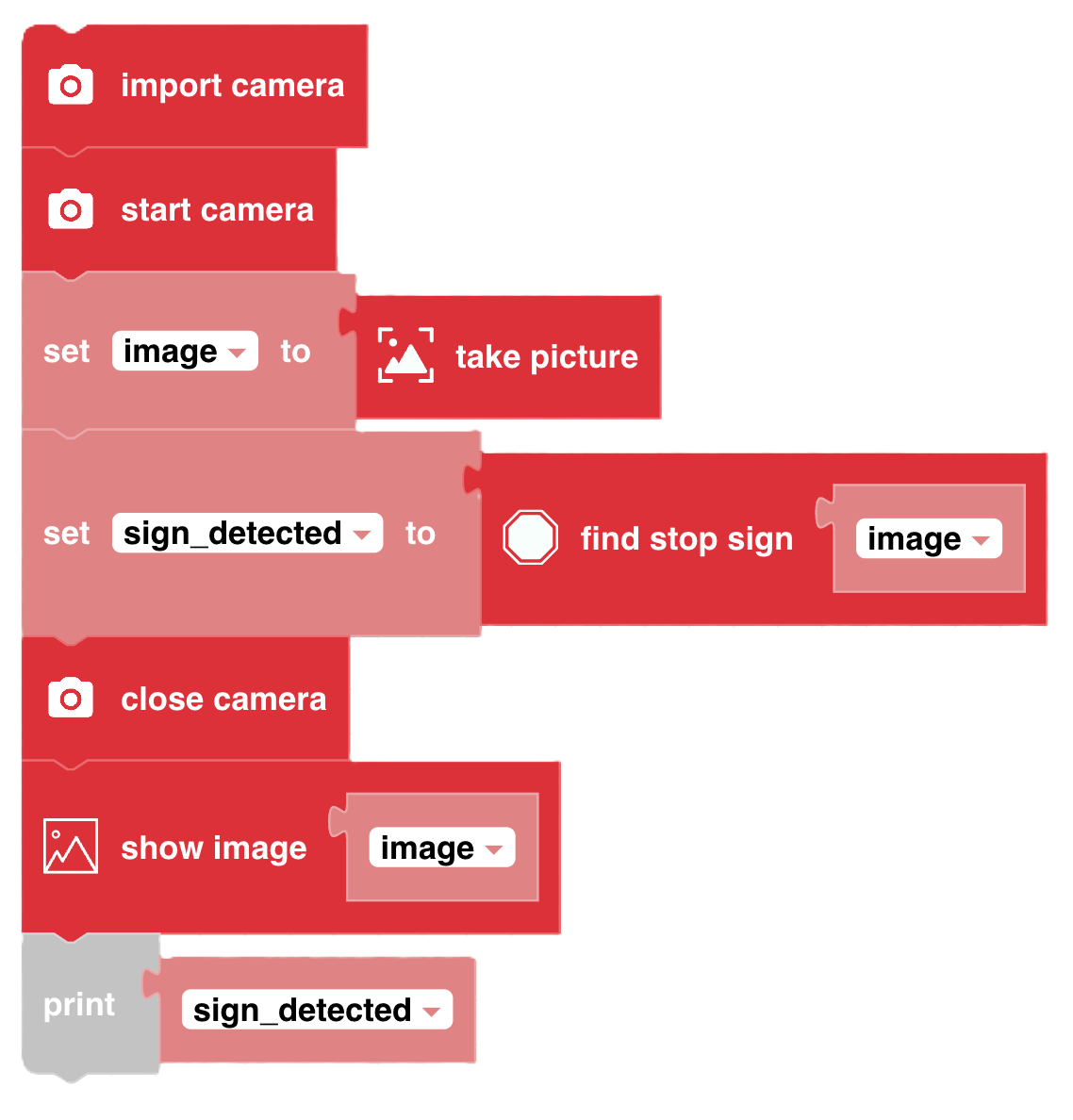
find face
Block
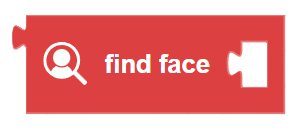
Description
Searches an image for a face. Returns True if a face was detected
Parameters
ndarray image: An image composed of a NumPy array of pixels.
Returns
boolean detection: Returns True if a face was detected. Otherwise, returns False.
Example
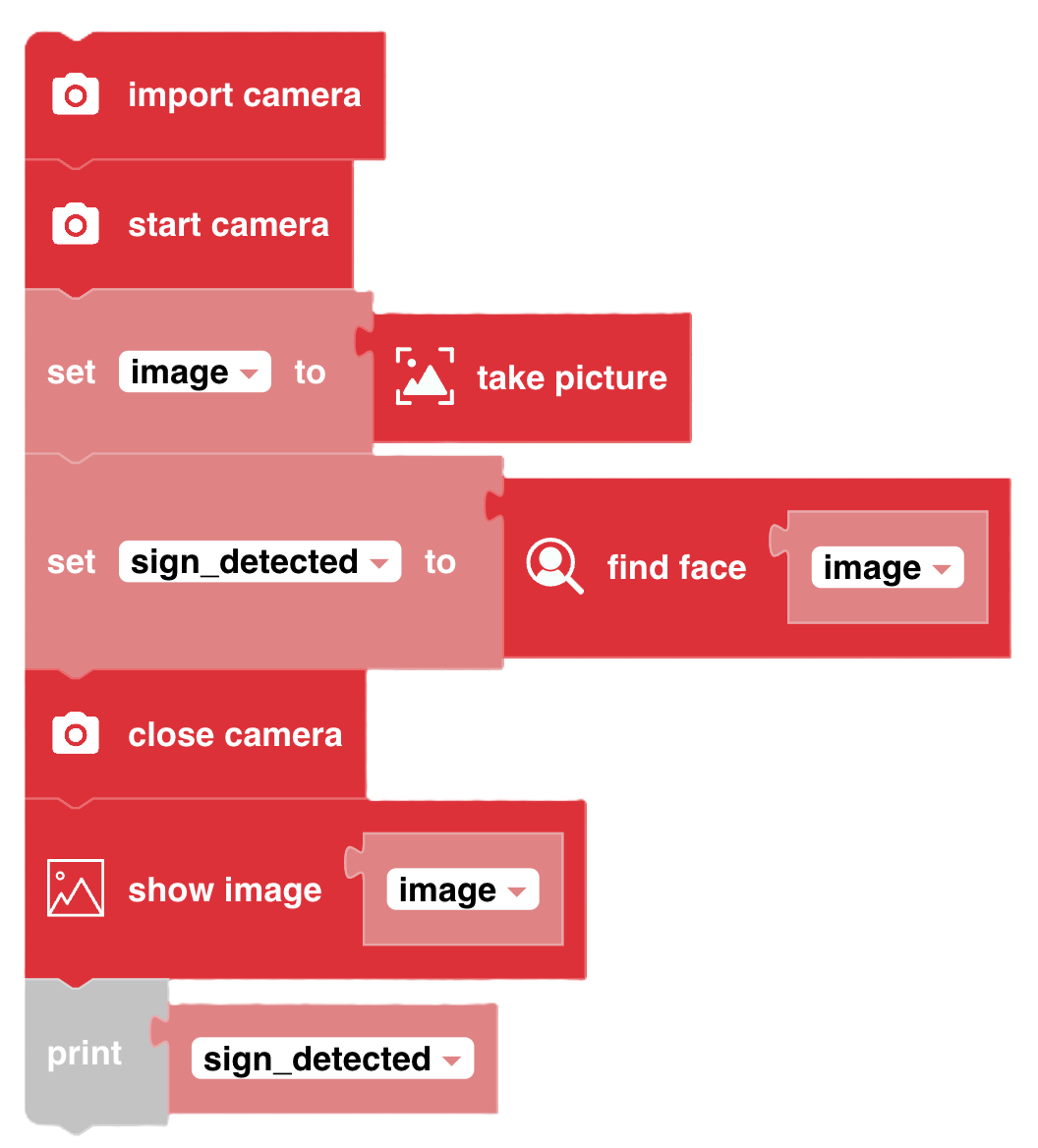
AI
prediction from frame
Block
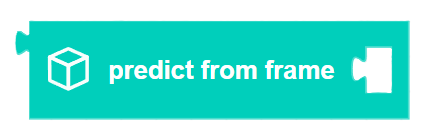
Description
Predicts a label from an image based on a previously trained color model. A KNN color model needs to be trained and loaded into the program to use predict_from_frame().
Parameters
ndarray image: An image composed of a NumPy array of pixels.
Returns
string label: The predicted label based on the image.
Example
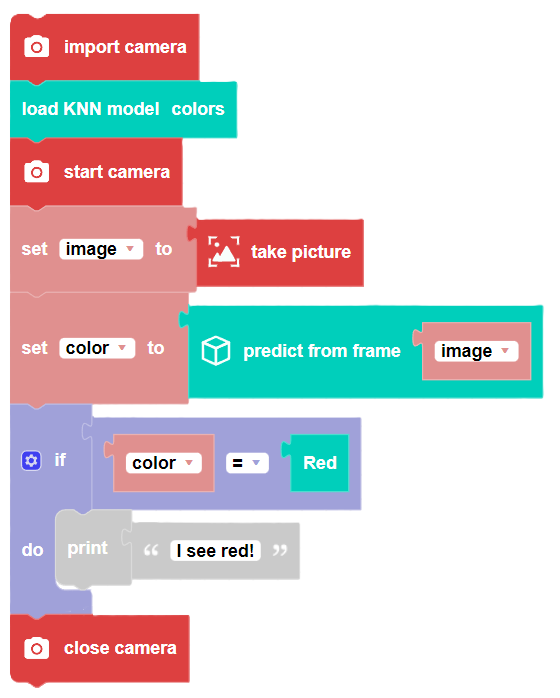
Lights
lights on
Block
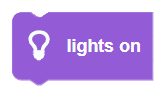
Description
Turns on both Zumi's headlights and brake lights.
Parameters
None
Returns
None
Example
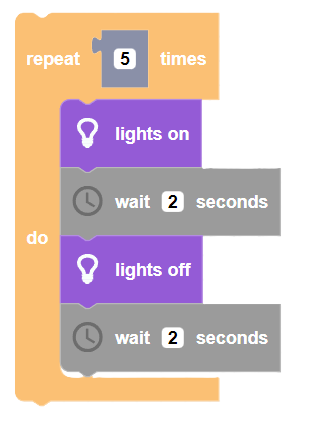
lights off
Block
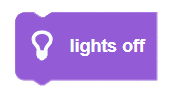
Description
Turns off both Zumi's headlights and brake lights.
Parameters
None
Returns
None
Example
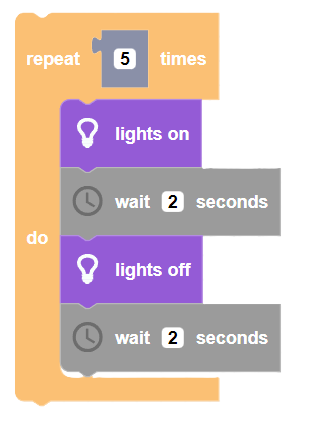
headlights on
Block
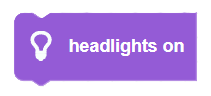
Description
Turns on Zumi's headlights.
Parameters
None
Returns
None
Example
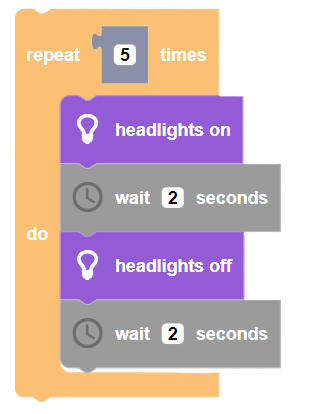
headlights off
Block
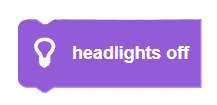
Description
Turns off Zumi's headlights.
Parameters
None
Returns
None
Example
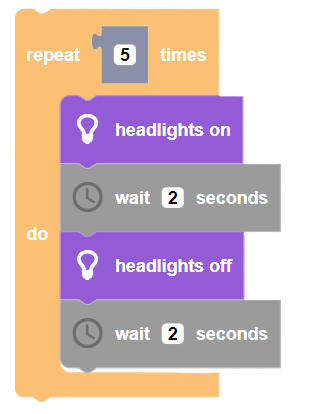
brake lights on
Block
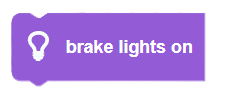
Description
Turns on Zumi's brake lights.
Parameters
None
Returns
None
Example
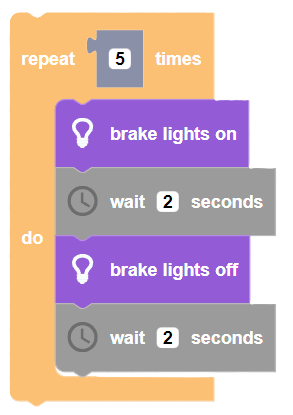
brake lights off
Block
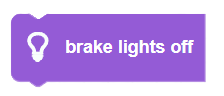
Description
Turns off Zumi's brake lights.
Parameters
None
Returns
None
Example
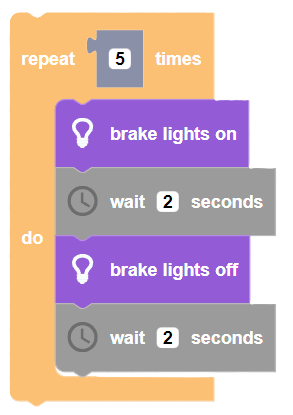
hazard lights on
Block
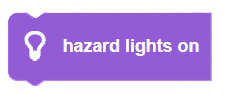
Description
Turns on Zumi's flashing hazard lights. They will flash indefinitely until turned off.
Parameters
None
Returns
None
Example
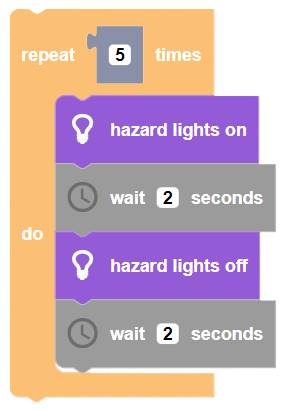
hazard lights off
Block
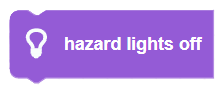
Description
Turns off Zumi's flashing hazard lights.
Parameters
None
Returns
None
Example
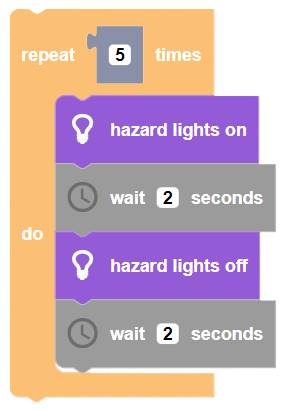
left signal on
Block
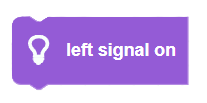
Description
Turns on Zumi's left turn signal. This function flashes Zumi's back left red LED until turned off.
Parameters
None
Returns
None
Example
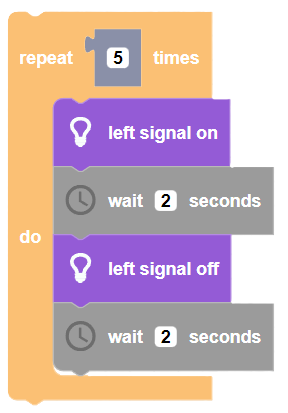
left signal off
Block
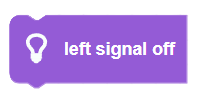
Description
Turns off Zumi's flashing left turn signal.
Parameters
None
Returns
None
Example
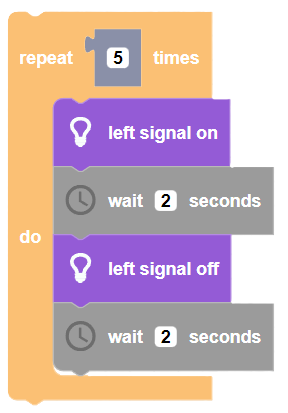
right signal on
Block
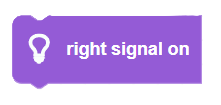
Description
Turns on Zumi's right turn signal. This function flashes Zumi's back right red LED until turned off.
Parameters
None
Returns
None
Example
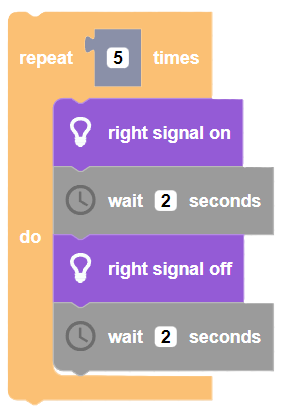
right signal off
Block
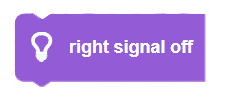
Description
Turns off Zumi's flashing right turn signal.
Parameters
None
Returns
None
Example
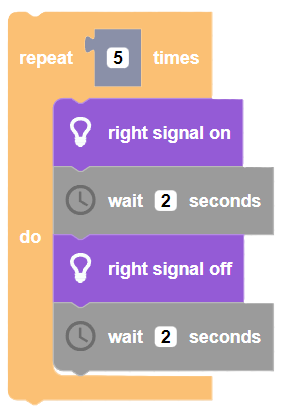