Senior Block Documentation
version 2.0.5 (Changelog)
Flight Commands
take off
Block

Description
Makes the drone take off.
Parameters
None
Returns
None
Example
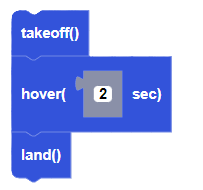
land
Block

Description
Makes the drone land.
Parameters
None
Returns
None
Example
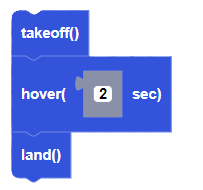
emergency stop
Block
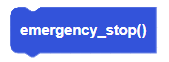
Description
Stops all commands to motors. The drone will stop flying immediately.
Parameters
None
Returns
None
Example
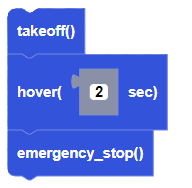
move([duration] seconds, [roll] %, [pitch] %, [yaw] %, [throttle] %)
Block
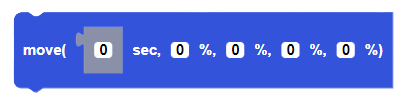
Description
Moves the drone for a certain amount of time (in seconds) in a given direction determined by the flight parameters.
Parameters
integer duration: the duration of the movement, in seconds
integer roll: roll power percentage between -100 and +100
integer pitch: pitch power percentage between -100 and +100
integer yaw: yaw power percentage between -100 and +100
integer throttle: throttle power percentage between -100 and +100
Returns
None
Example
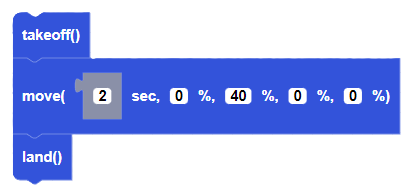
turn([direction] , [duration] seconds, [power] %)
Block
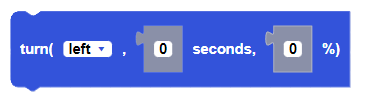
Description
Turns CoDrone Mini left or right for a duration in seconds and at a power percentage from 0 to 100% speed.
Parameters
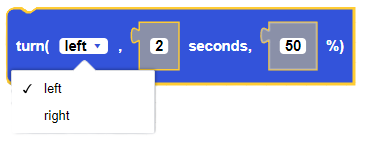
direction: left, right
integer duration: the duration of turn in seconds
integer power: the power of the turn from 0 to 100
Returns
None
Example
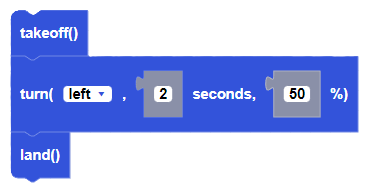
hover([seconds] sec)
Block
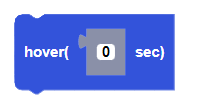
Description
This function makes the drone hover for a duration in seconds.
Parameters
integer duration: the duration of the hovering in seconds
Returns
None
Example
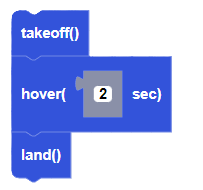
Flight Variables
set_roll()
Block
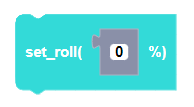
Description
This function sets the roll direction variable but will not send a move command. Negative values will move the drone to the left and positive values will move the drone to the right.
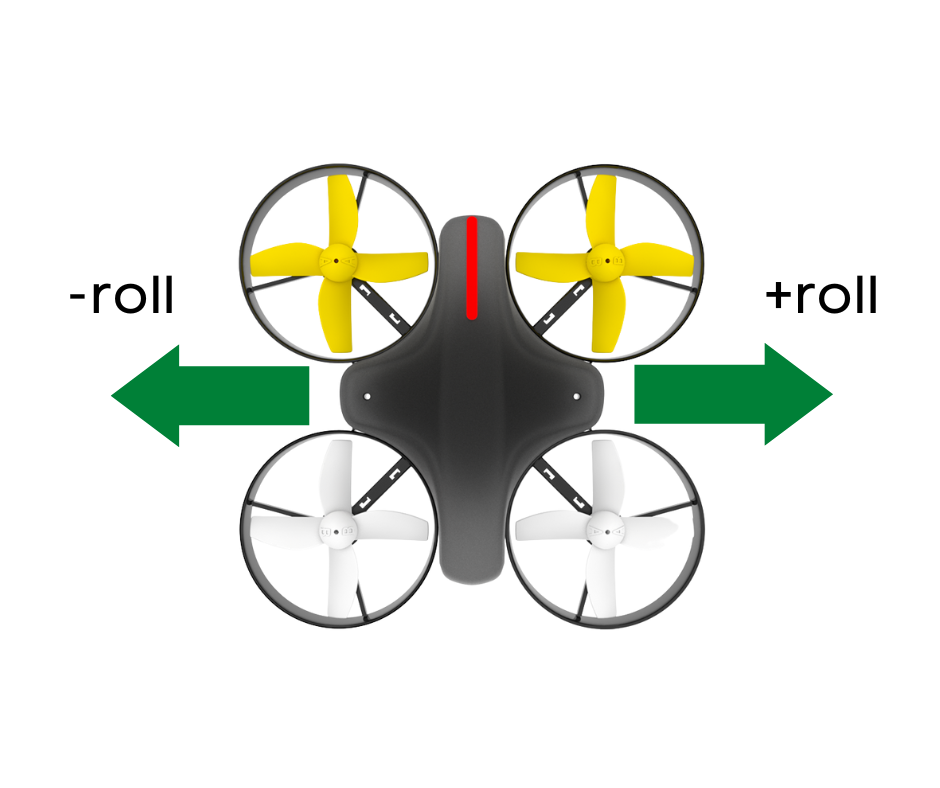
Parameters
integer power: the power of the roll movement between -100 and 100
Returns
None
Exmaple
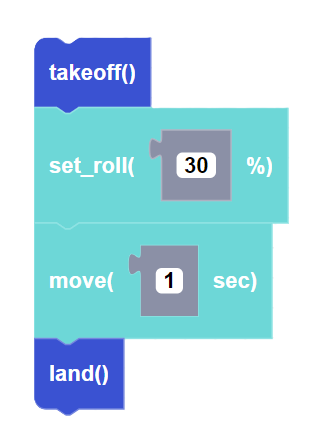
set_pitch()
Block
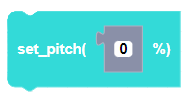
Description
This function sets the pitch direction variable but will not send a move command. Negative values will move the drone backward and positive values will move the drone forward.
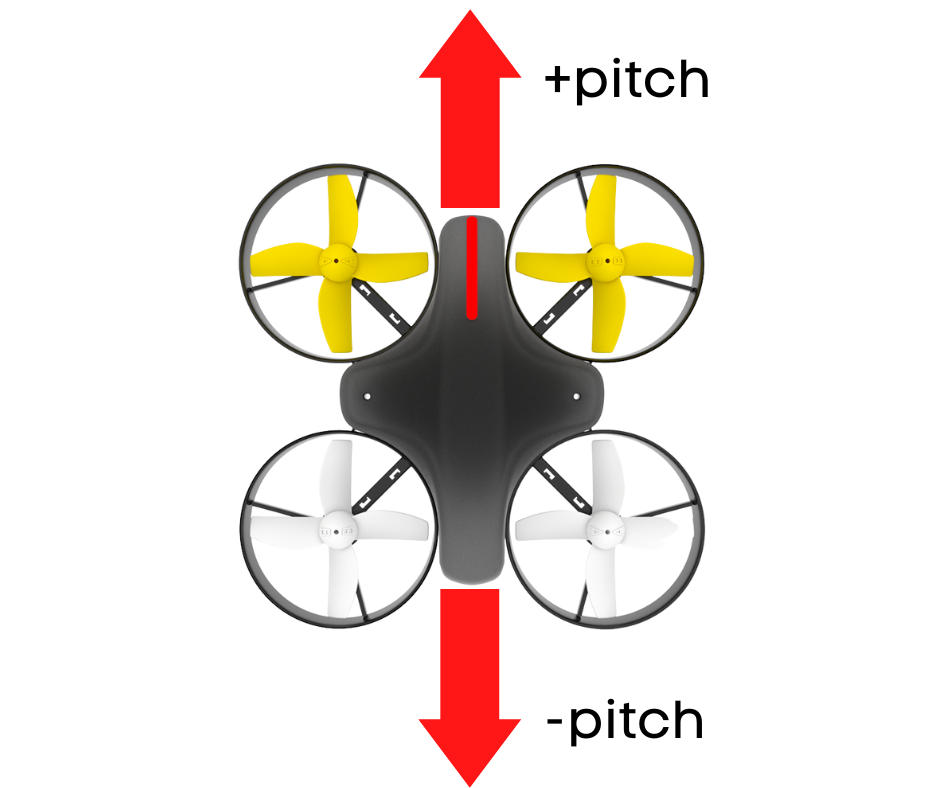
Parameters
integer power: the power of the pitch movement between -100 and 100
Returns
None
Example
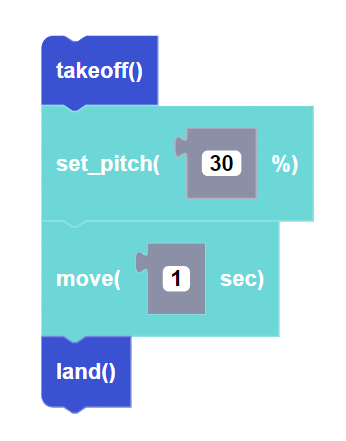
set_yaw()
Block
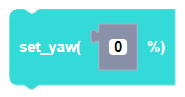
Description
This function sets the yaw direction variable but will not send a move command. Negative values will turn the drone to the right and positive values will turn the drone to the left.
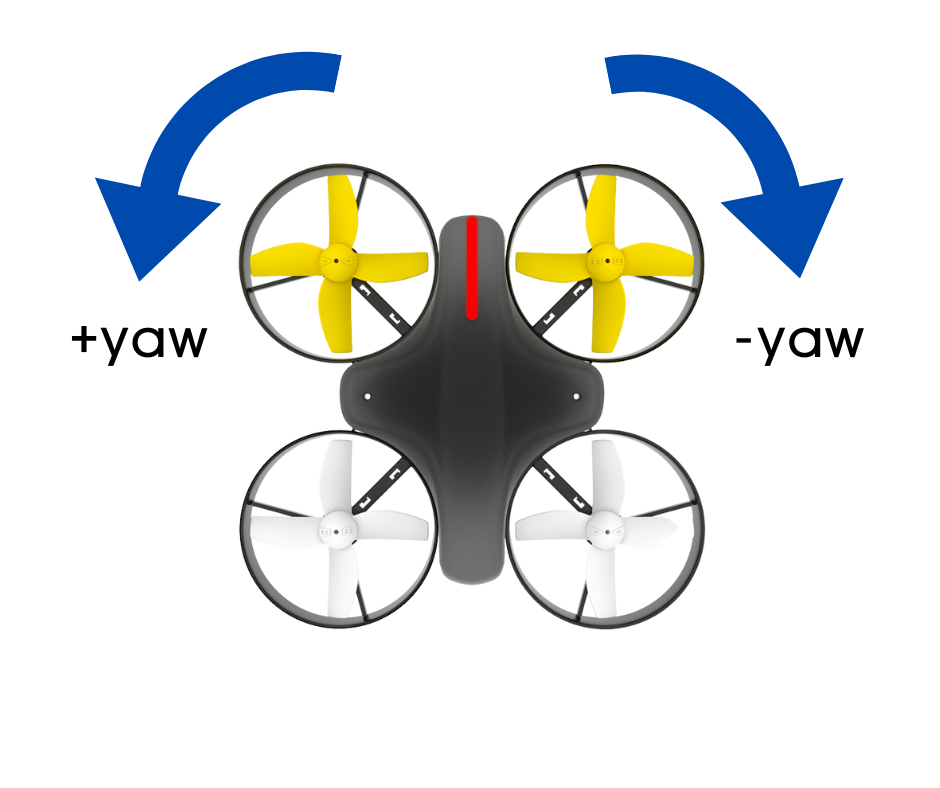
Parameters
integer power: the power of the yaw movement between -100 and 100
Returns
None
Example
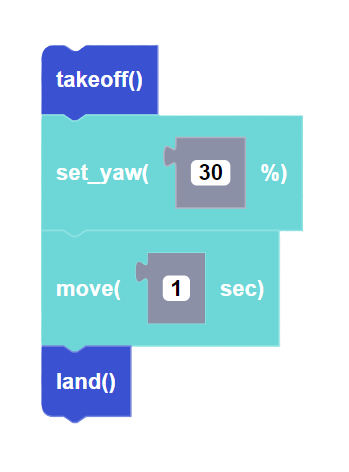
set_throttle()
Block
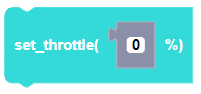
Description
This function sets the throttle direction variable but will not send a move command. Negative values will move the drone downward and positive values will move the drone upward.
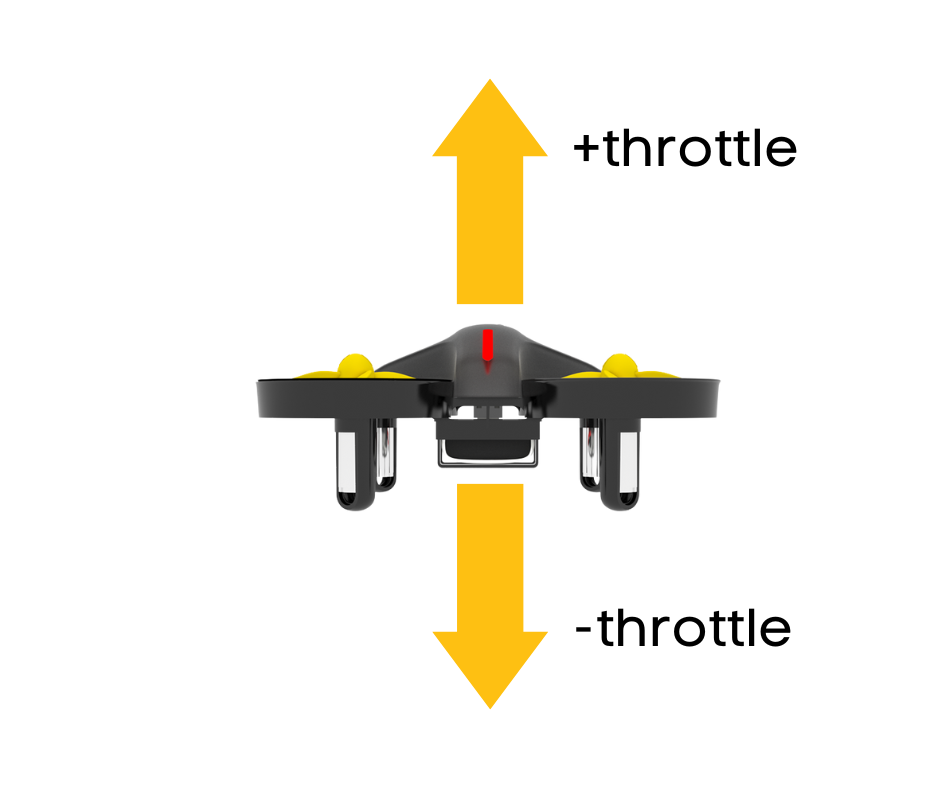
Parameters
integer power: the power of the throttle movement between -100 and 100
Returns
None
Example
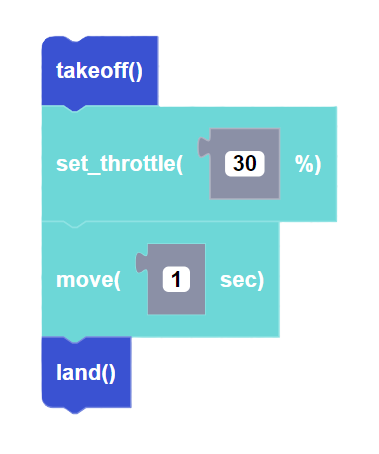
move(duration)
Block
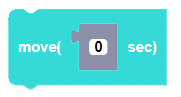
Description
Moves CoDrone Mini for a duration in seconds in the direction set by the flight variables.
Parameters
integer duration: the duration of movement in seconds
Returns
None
Example
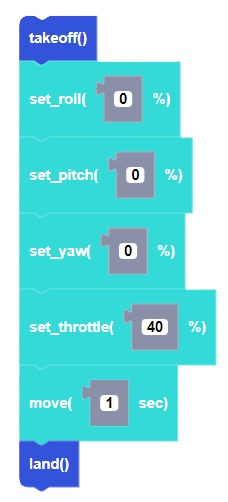
move()
Block
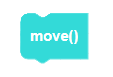
Description
Moves CoDrone Mini in the direction set by the flight variables with the smallest duration possible (about 0.01 seconds). Since it has no specified duration, it is often used inside of a loop to check sensors simultaneously.
Parameters
None
Returns
None
Example
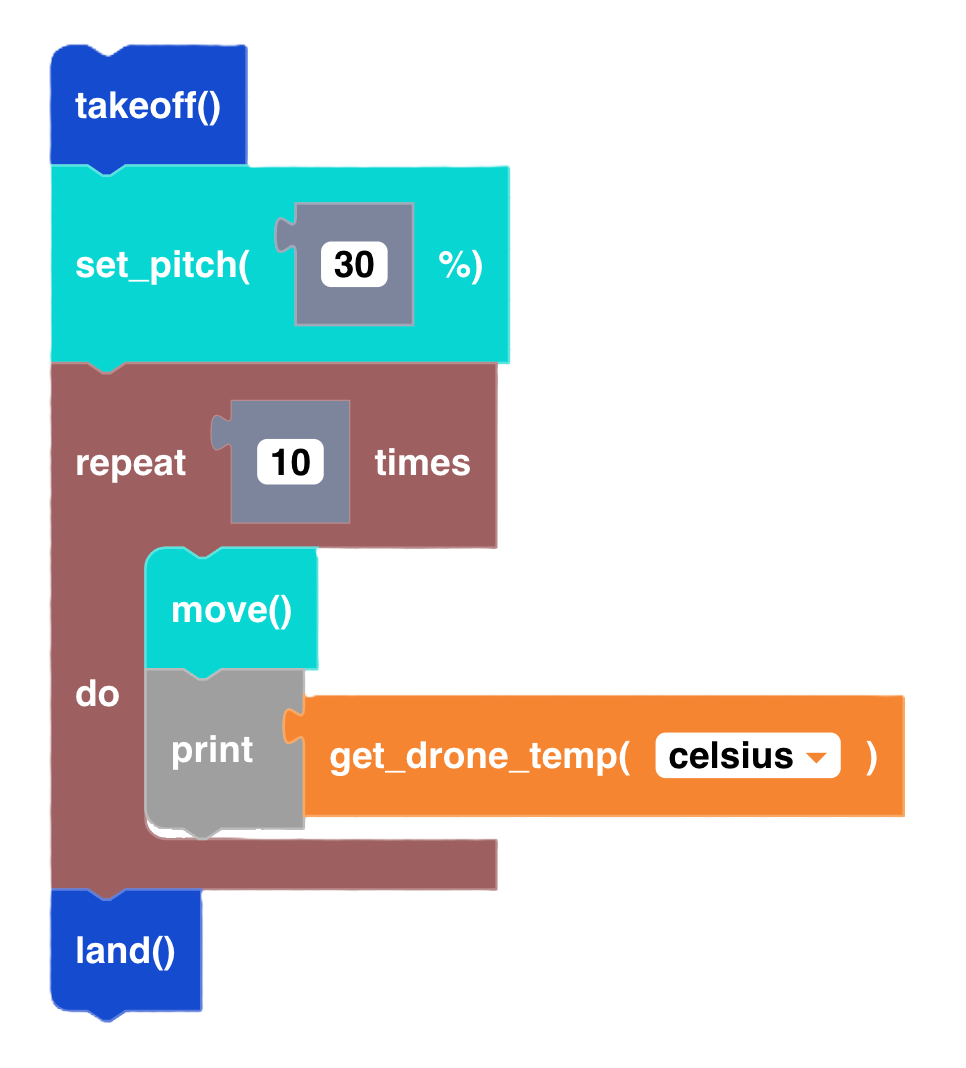
set_trim()
Block
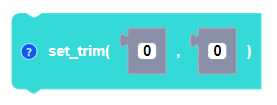
Description
Sets the trim values to adjust for any drifting while CoDrone Mini is flying. Set the trim values in the opposite direction of drift. For example, if the drone is drifting to the right, set roll to a negative value.
Parameters
integer roll: the power of the roll movement between -100 and 100
integer pitch: the power of the pitch movement between -100 and 100
Returns
None
Example
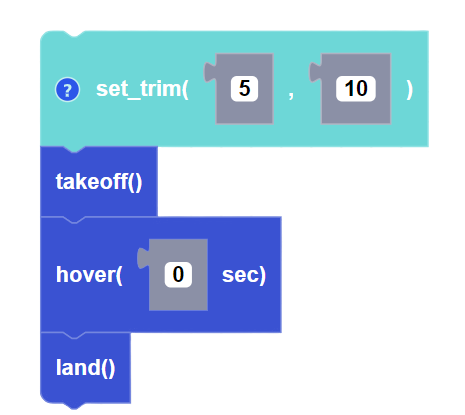
get_trim()
Block
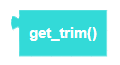
Description
Returns the current trim values. Combine with a print statement to see the results printed to the console.
Parameters
None
Returns
integer roll: the power of the roll movement between -100 and 100
integer pitch: the power of the pitch movement between -100 and 100
Example
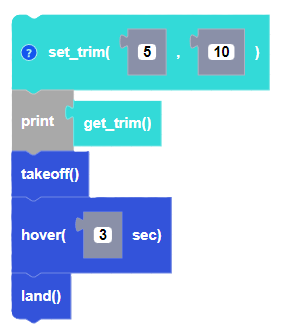
reset_trim()
Block
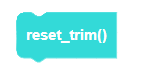
Description
Resets the trim values to (0,0).
Parameters
None
Returns
None
Example
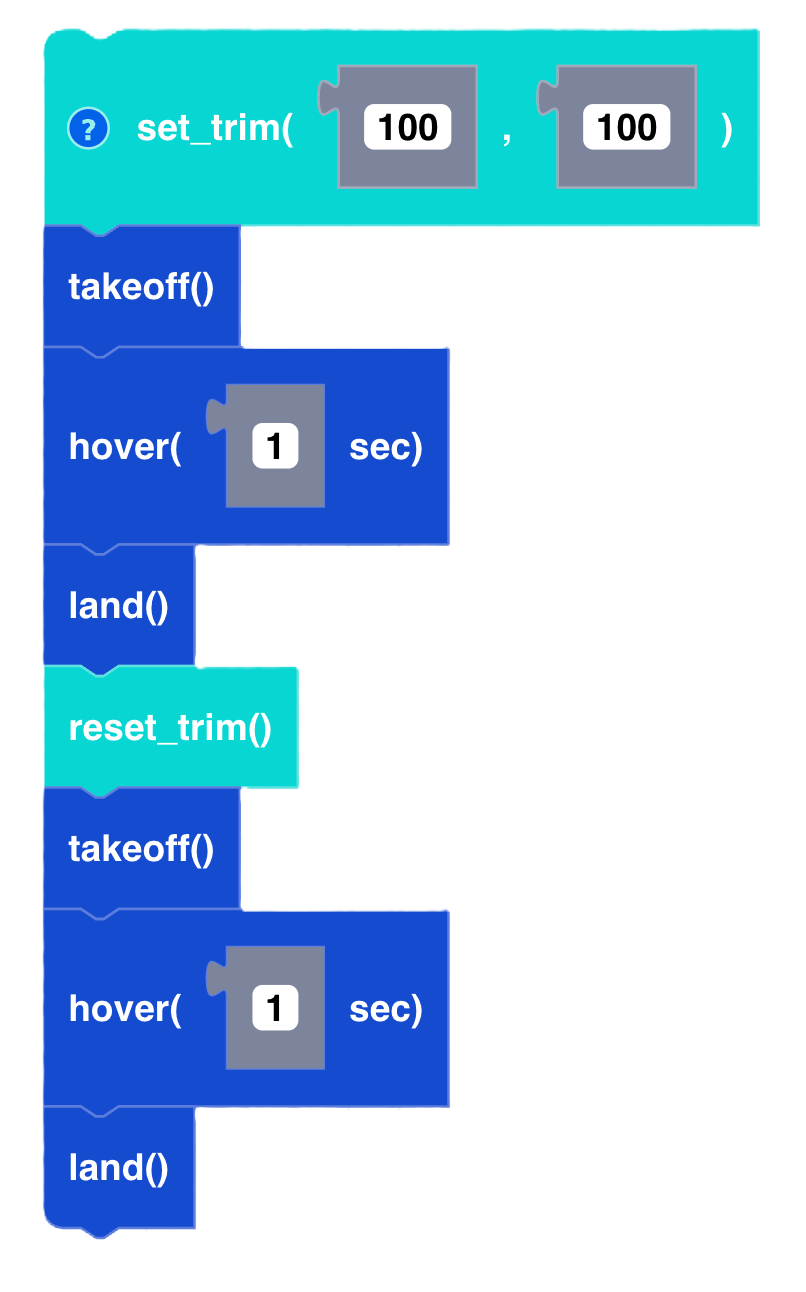
get_roll()
Block
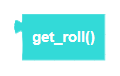
Description
Returns the current value for the roll flight variable.
Parameters
None
Returns
integer roll: the power of the roll movement between -100 and 100
Example
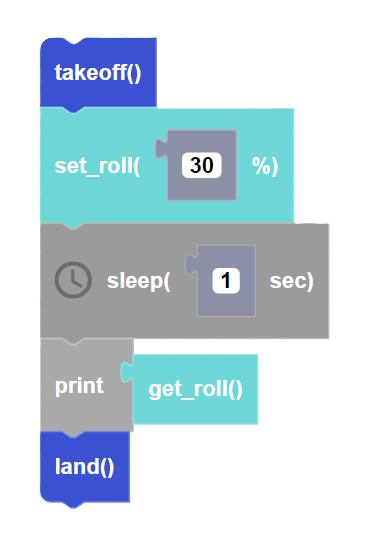
get_pitch()
Block
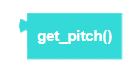
Description
Returns the current value for the pitch flight variable.
Parameters
None
Returns
integer pitch: the power of the pitch movement between -100 and 100
Example
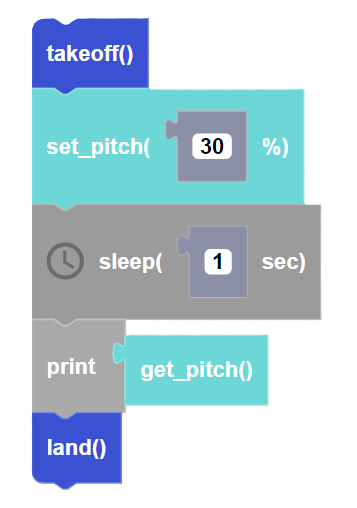
get_yaw()
Block
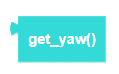
Description
Returns the current value for the yaw flight variable.
Parameters
None
Returns
integer yaw: the power of the yaw movement between -100 and 100
Example
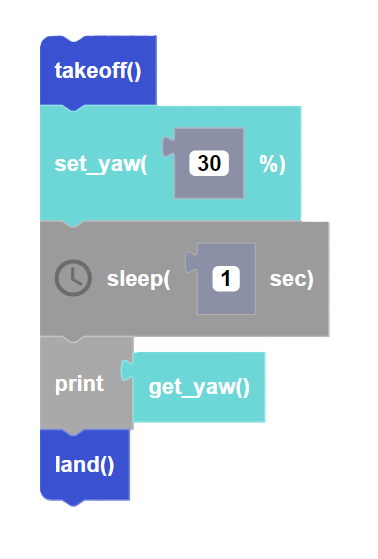
get_throttle()
Block
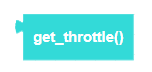
Description
Returns the current value for the throttle flight variable.
Parameters
None
Returns
integer throttle: the power of the throttle movement between -100 and 100
Example
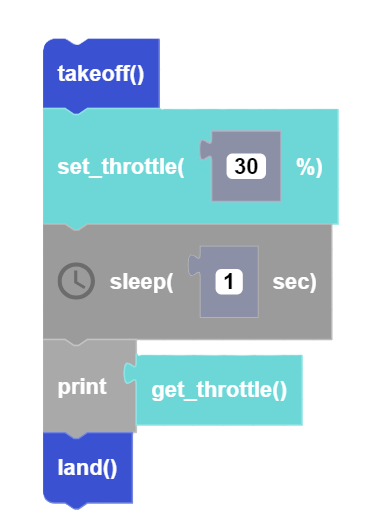
Status Checkers
code_is_running()
Block
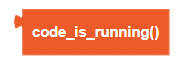
Description
Returns a True value while your code is running. Use this block instead of "while True" when you want to run a "forever" loop. Use the "Stop" button in Blockly to stop the program.
Parameters
None
Returns
boolean running: Returns True if program is running, False if user presses "Stop"
Example
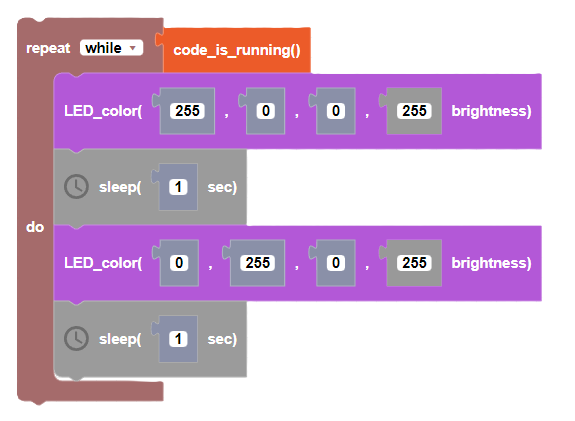
Sensors
get_angle()
Block
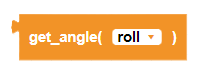
Description
This function returns the roll, pitch, and yaw angles from the gyroscope. The 0 angle is set when pairing.
Parameters
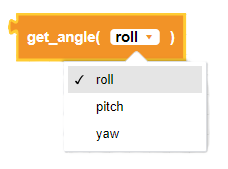
axis: select roll, pitch or yaw
Returns
integer angle: angle, in degrees, from the starting position
Example
For this example, manually turn your drone by hand to see the yaw angle change.
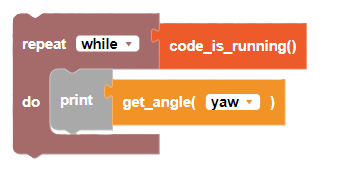
get_angle()
Block
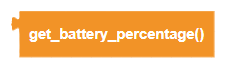
Description
This function returns the current battery percentage of the drone battery.
Parameters
None
Returns
integer percentage: the battery percentage from 0 to 100
Example
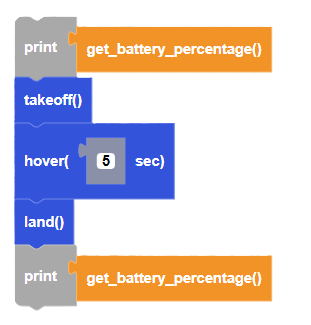
get_drone_temp()
Block
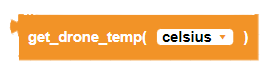
Description
This block returns the current temperature of the drone in either Celsius or Fahrenheit.
Parameters
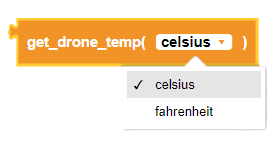
Returns
float temperature: Temperature of the drone, either Celsius or Fahrenheit
Example
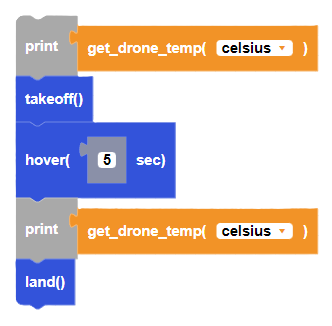
get_height()
Block
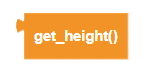
Description
This function returns the current estimated height of the drone from the surface at the moment of pairing. This is calculated by the barometer (air pressure sensor).
Parameters
None
Returns
float height: the height of the drone in centimeters
Example
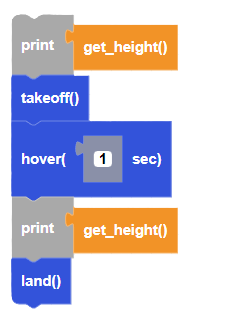
get_pressure()
Block
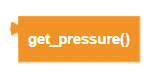
Description
This function returns barometer data in Pascals.
Note: 1atm = 101325 Pa
Parameters
None
Returns
float pressure: the pressure measured from barometer in Pascals
Example
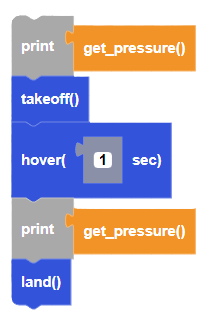
reset_sensor()
Block
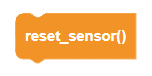
Description
Resets the gyroscope angles to 0. The drone will flash while the gyroscope is recalibrating. Do not handle or move the drone during calibration.
Parameters
None
Returns
None
Example
Rotate the drone with your hand on a flat surface. See the angles change by observing the printed values in the console. Stop moving the drone when the screen prints "resetting". After resetting, the gyroscope angles will return to 0.
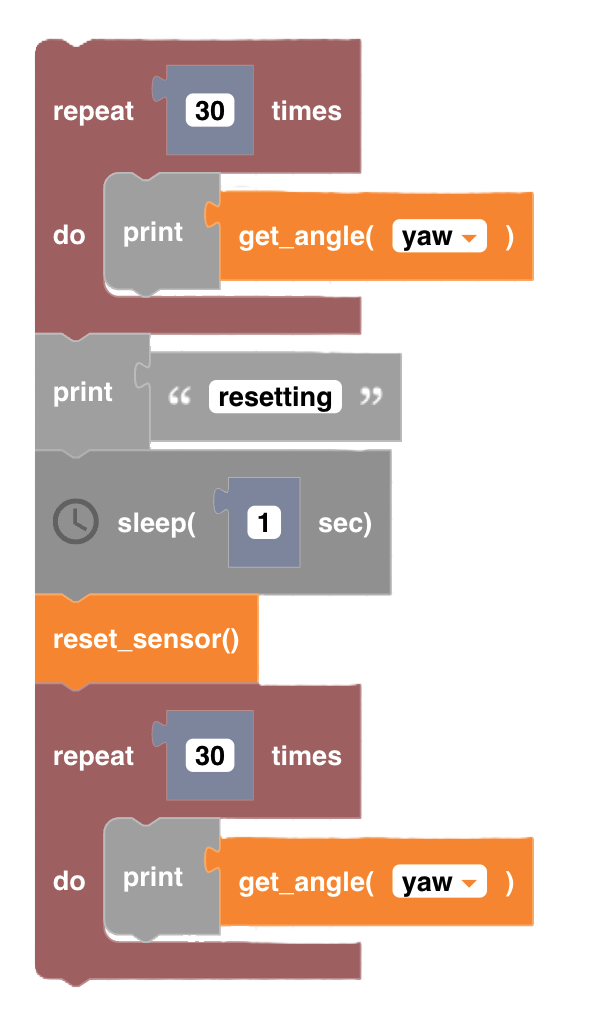
Lights
LED_color()
Block
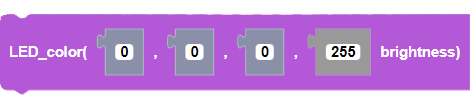
Description
Sets the color of CoDrone Mini's LED. Colors are set by using its RGB (red, green, blue) equivalent values.
Parameters
integer red: the pixel value for the color red between 0 and 255
integer green: the pixel value for the color green between 0 and 255
integer blue: the pixel value for the color blue between 0 and 255
integer brightness: the brightness of the LEDs between 0 and 255
Returns
None
Example
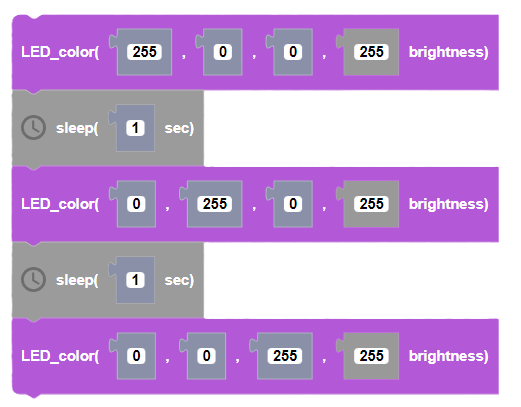
LED_color(r,g,b,brightness)
Block
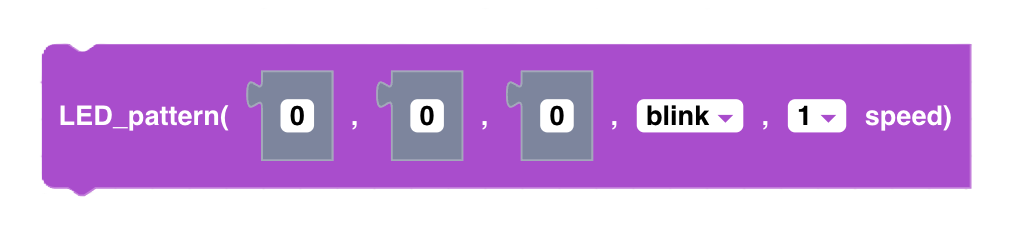
Description
Sets the color of CoDrone Mini's LED blinking pattern. "Blink" flashes the LED on and off and an interval determined by the speed parameter. "Double blink" flashes the LED twice before pausing at the interval determined by the speed parameter.
Parameters
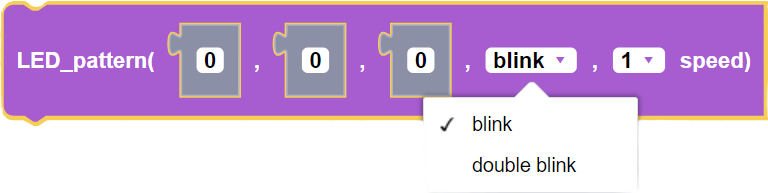
integer red: the pixel value for the color red between 0 and 255
integer green: the pixel value for the color green between 0 and 255
integer blue: the pixel value for the color blue between 0 and 255
pattern: blink or double blink
integer speed: the speed of the lighting pattern from 1 to 10
Returns
None
Example
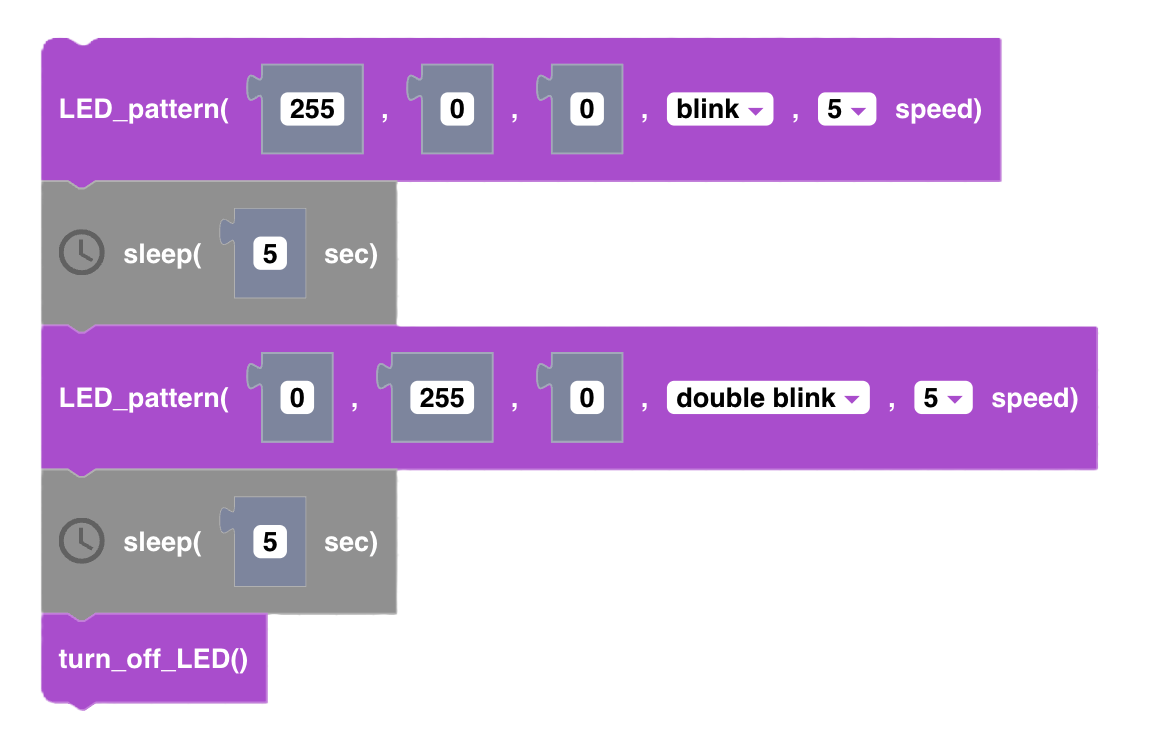
turn_off_LED()
Block
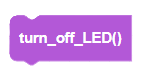
Description
Turns off CoDrone Mini's LED.
Parameters
None
Returns
None
Example
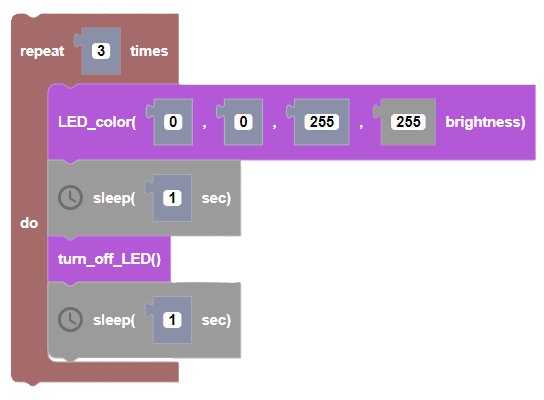
reset_LED()
Block
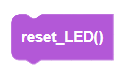
Description
Reset's the Codrone mini's LED to its default state (solid red LED).
Parameters
None
Returns
None
Example
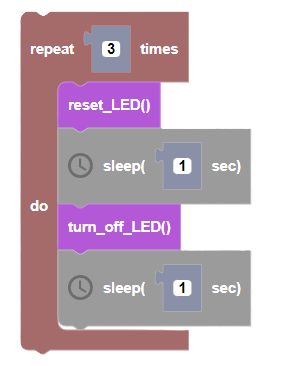
Sound
play_note(note, [duration] seconds)
Block
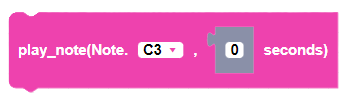
Description
Plays a note for a specified amount of time using the controller buzzer.
Parameters
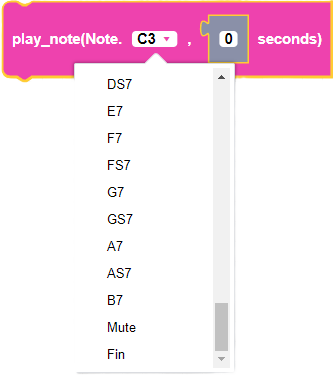
note: note from C3 to B7
integer duration: the duration of the buzzer note, in seconds
Returns
None
Example
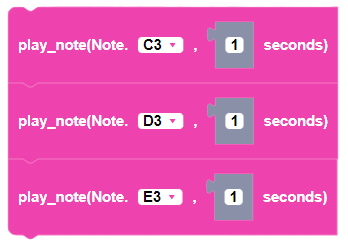
play_note([frequency] Hertz, [duration] seconds)
Block
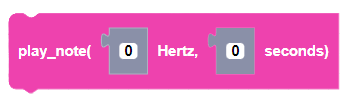
Description
Plays a note at a particular frequency for a specified amount of time using the controller buzzer.
Parameters
integer frequency: The note's frequency in Hertz
integer duration: the duration of the note played in seconds
Returns
None
Example
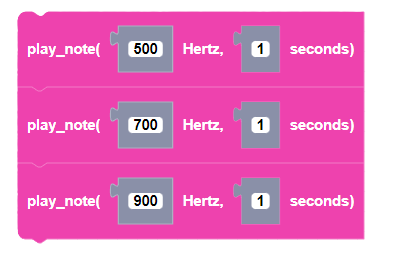